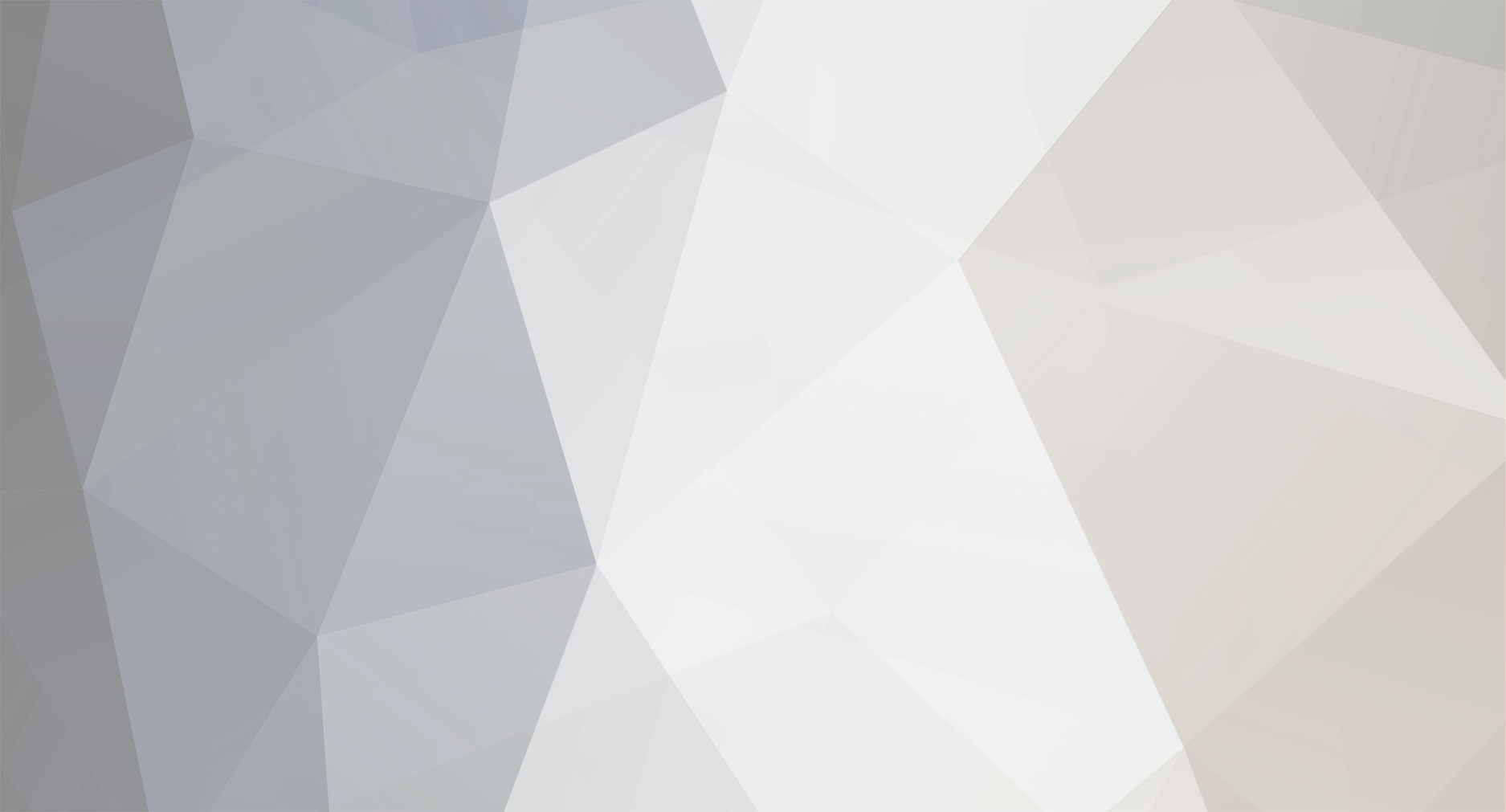
leo1177
-
Posts
208 -
Joined
-
Last visited
-
Days Won
2
Content Type
Profiles
Forums
Articles
Posts posted by leo1177
-
-
-
hi this is a demo version, can someone change to a fullversion?
-
it is the same
-
i think this indicators makes very bad the supply/demand zones it is a lot of better do that by himself....
-
can someone change this formula
#region Using declarations
using System;
using System.ComponentModel;
using System.Diagnostics;
using System.Drawing;
using System.Drawing.Drawing2D;
using System.Xml.Serialization;
using NinjaTrader.Cbi;
using NinjaTrader.Data;
using NinjaTrader.Gui.Chart;
#endregion
// This namespace holds all indicators and is required. Do not change it.
namespace NinjaTrader.Indicator
{
/// <summary>
/// Enter the description of your new custom indicator here
/// </summary>
[Description("Enter the description of your new custom indicator here")]
public class LWADMA : Indicator
{
#region Variables
private int perioda = 3, periodb = 10;
private double trueRangeHigh;
private double trueRangeLow;
private double priceMove;
private DataSeries iAD;
#endregion
/// <summary>
/// This method is used to configure the indicator and is called once before any bar data is loaded.
/// </summary>
protected override void Initialize()
{
Add(new Plot(new Pen(Color.Blue, 3), "AD")); // plot 0
trueRangeHigh = trueRangeLow = priceMove = 0d;
iAD = new DataSeries(this);
Overlay = false;
PriceTypeSupported = true;
}
/// <summary>
/// Called on each bar update event (incoming tick)
/// </summary>
protected override void OnBarUpdate()
{
// Use this method for calculating your indicator values. Assign a value to each
// plot below by replacing 'Close[0]' with your own formula.
if (CurrentBar >0)
{
// calculate true range high and low
trueRangeHigh = Math.Max(High[0], Close[1]);
trueRangeLow = Math.Min(Low[0], Close[1]);
priceMove = 0;
// compare closing price to yesterdays
// CumulativeSum (Iff (Close > Close. 1 , Close - True Low, Iff (Close < Close A , Close - True High,0)))
if(Close[0] > Close[1])
{
priceMove = Close[0] - trueRangeLow;
}
else if(Close[0] < Close[1])
{
priceMove = Close[0] - trueRangeHigh;
}
else if(Close[0] == Close[1])
{
priceMove = 0d;
}
iAD.Set((CurrentBar == 0 ? (Close[0] - Low[0]) : iAD[1]) + priceMove);
}
// ((MovingAvgMethod (Williams AccumDist Positive , vara , Method) -
// MovingAvgMethod (Williams AccumDist Positive , varb , Method)) /
// MovingAvgMethod (Williams AccumDist Positive , vara , Method)) * 100
if (CurrentBar > periodb)
{
AD.Set((SMA(iAD, perioda)[0] - SMA(iAD, periodb)[0])/SMA(iAD, perioda)[0]*100);
}
}
#region Properties
[browsable(false)] // this line prevents the data series from being displayed in the indicator properties dialog, do not remove
[XmlIgnore()] // this line ensures that the indicator can be saved/recovered as part of a chart template, do not remove
public DataSeries AD
{
get { return Values[0]; }
}
[Description("Numbers of bars used for calculations")]
[GridCategory("Parameters")]
public int PeriodA
{
get { return perioda; }
set { perioda = Math.Max(1, value); }
}
[Description("Numbers of bars used for calculations")]
[GridCategory("Parameters")]
public int PeriodB
{
get { return periodb; }
set { periodb = Math.Max(1, value); }
}
#endregion
}
}
#region NinjaScript generated code. Neither change nor remove.
// This namespace holds all indicators and is required. Do not change it.
namespace NinjaTrader.Indicator
{
public partial class Indicator : IndicatorBase
{
private LWADMA[] cacheLWADMA = null;
private static LWADMA checkLWADMA = new LWADMA();
/// <summary>
/// Enter the description of your new custom indicator here
/// </summary>
/// <returns></returns>
public LWADMA LWADMA(int periodA, int periodB)
{
return LWADMA(Input, periodA, periodB);
}
/// <summary>
/// Enter the description of your new custom indicator here
/// </summary>
/// <returns></returns>
public LWADMA LWADMA(Data.IDataSeries input, int periodA, int periodB)
{
if (cacheLWADMA != null)
for (int idx = 0; idx < cacheLWADMA.Length; idx++)
if (cacheLWADMA[idx].PeriodA == periodA && cacheLWADMA[idx].PeriodB == periodB && cacheLWADMA[idx].EqualsInput(input))
return cacheLWADMA[idx];
lock (checkLWADMA)
{
checkLWADMA.PeriodA = periodA;
periodA = checkLWADMA.PeriodA;
checkLWADMA.PeriodB = periodB;
periodB = checkLWADMA.PeriodB;
if (cacheLWADMA != null)
for (int idx = 0; idx < cacheLWADMA.Length; idx++)
if (cacheLWADMA[idx].PeriodA == periodA && cacheLWADMA[idx].PeriodB == periodB && cacheLWADMA[idx].EqualsInput(input))
return cacheLWADMA[idx];
LWADMA indicator = new LWADMA();
indicator.BarsRequired = BarsRequired;
indicator.CalculateOnBarClose = CalculateOnBarClose;
#if NT7
indicator.ForceMaximumBarsLookBack256 = ForceMaximumBarsLookBack256;
indicator.MaximumBarsLookBack = MaximumBarsLookBack;
#endif
indicator.Input = input;
indicator.PeriodA = periodA;
indicator.PeriodB = periodB;
Indicators.Add(indicator);
indicator.SetUp();
LWADMA[] tmp = new LWADMA[cacheLWADMA == null ? 1 : cacheLWADMA.Length + 1];
if (cacheLWADMA != null)
cacheLWADMA.CopyTo(tmp, 0);
tmp[tmp.Length - 1] = indicator;
cacheLWADMA = tmp;
return indicator;
}
}
}
}
// This namespace holds all market analyzer column definitions and is required. Do not change it.
namespace NinjaTrader.MarketAnalyzer
{
public partial class Column : ColumnBase
{
/// <summary>
/// Enter the description of your new custom indicator here
/// </summary>
/// <returns></returns>
[Gui.Design.WizardCondition("Indicator")]
public Indicator.LWADMA LWADMA(int periodA, int periodB)
{
return _indicator.LWADMA(Input, periodA, periodB);
}
/// <summary>
/// Enter the description of your new custom indicator here
/// </summary>
/// <returns></returns>
public Indicator.LWADMA LWADMA(Data.IDataSeries input, int periodA, int periodB)
{
return _indicator.LWADMA(input, periodA, periodB);
}
}
}
// This namespace holds all strategies and is required. Do not change it.
namespace NinjaTrader.Strategy
{
public partial class Strategy : StrategyBase
{
/// <summary>
/// Enter the description of your new custom indicator here
/// </summary>
/// <returns></returns>
[Gui.Design.WizardCondition("Indicator")]
public Indicator.LWADMA LWADMA(int periodA, int periodB)
{
return _indicator.LWADMA(Input, periodA, periodB);
}
/// <summary>
/// Enter the description of your new custom indicator here
/// </summary>
/// <returns></returns>
public Indicator.LWADMA LWADMA(Data.IDataSeries input, int periodA, int periodB)
{
if (InInitialize && input == null)
throw new ArgumentException("You only can access an indicator with the default input/bar series from within the 'Initialize()' method");
return _indicator.LWADMA(input, periodA, periodB);
}
}
}
#endregion
to this one
(((MovingAvgMethod (Close , 3 , 1) - MovingAvgMethod (Close , 10 , 1)) / MovingAvgMethod (Close , 3 , 1)) * 100 + ((MovingAvgMethod (Williams AccumDist Positive , 3 , 1) - MovingAvgMethod (Williams AccumDist Positive , 9 , 1)) / MovingAvgMethod (Williams AccumDist Positive , 3 , 1)) * 100) / 2
Williams AccumDist Positive
Williams AccumDist + (100 - Lowest (Williams AccumDist , 0))
Williams AccumDist
CumulativeSum (Iff (Close > Close.1 , Close - True Low , Iff (Close < Close.1 , Close - True High , 0)))
-
thx for help
-
Indo-Invastasi is the wrong passwort
-
no why? it is the same... only charttrader is active
-
01.04 april april
-
http://www.ireallytrade.com/artshorttermtrading.html
hi, have someone stuff about this course from larry?
[-O<[-O<[-O<
-
hey codrut, do you have some more stuff from larry? maybe the last course? if yes, please share...
-
- codrut_8, ⭐ SignalTime, ⭐ Billee and 17 others
-
20
-
gani84
i know you have this course, please share with orther people...
it is not correct, use this forum for yourself and nothing giving back!
-
i don`t need because i use kinetick end of day datafeed :)
-
great kyrus thank you!!!!
-
no sorry.........
-
can someone reupload please
-
-
-
have someone this course from larry williams please?
-
you right, for 3 month 150 dollar you can pay andrey my russian brother or pay ninjatrader 50 dollar every month lol
-
can someone make the emu without hardware id? please
-
thanks kishisaki
-
lol.........
Larry Williams
in Ninja Trader 7
Posted