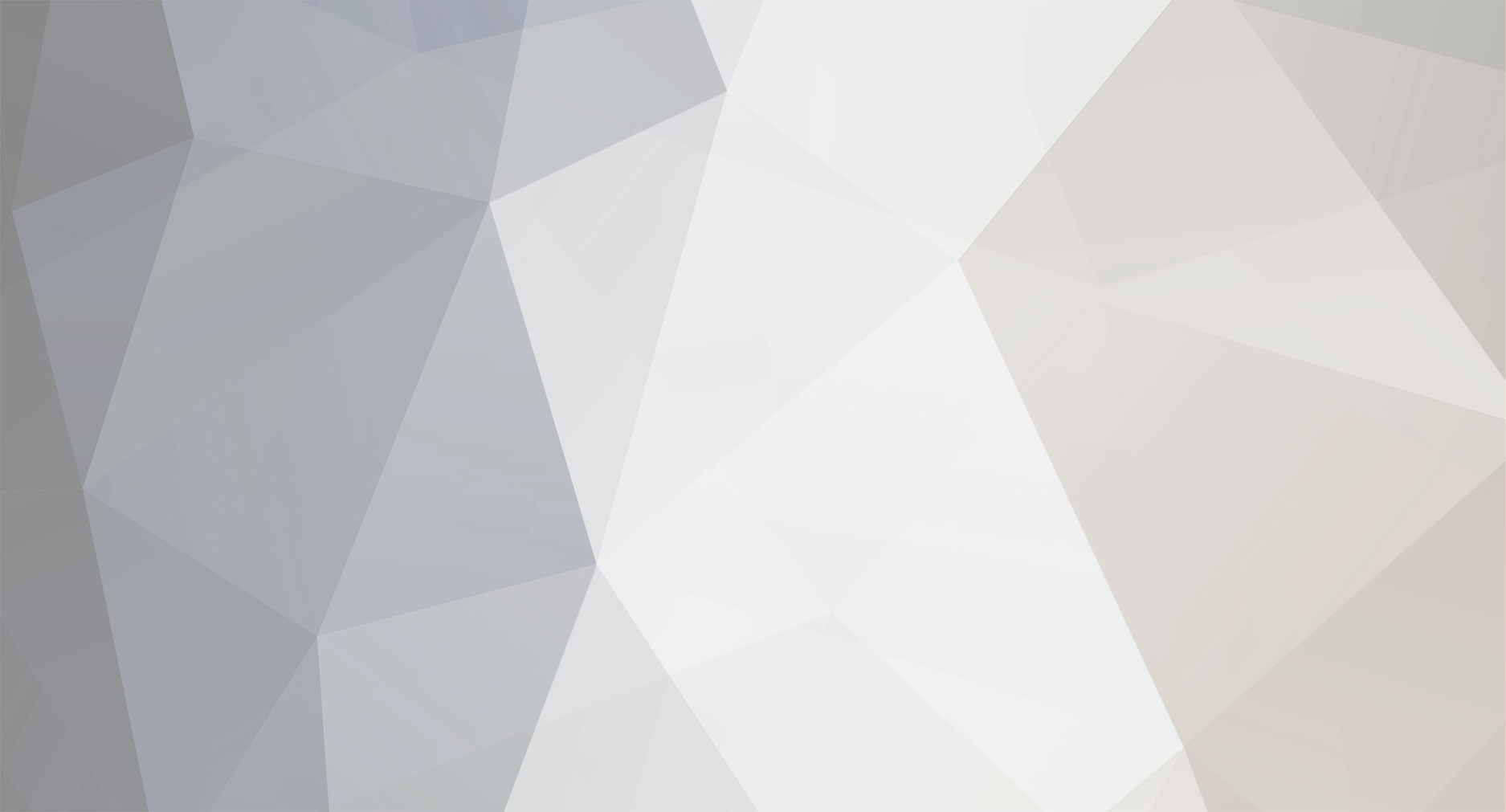
udc
-
Posts
224 -
Joined
-
Last visited
-
Days Won
24
Content Type
Profiles
Forums
Articles
Posts posted by udc
-
-
NMR for MT4
NMR, a.k.a. natural market river.
There is a funny moment in the video where Pat first says "we are dividing...", camera cut and the next moment he is saying "we are actually multiplying rather than dividing..." :) Those people sitting in the seminar must have had fun.
Original NMR on TradeStation:
http://img818.imageshack.us/img818/8089/nmrtradestation.png
NMR on Metatrader:
http://img812.imageshack.us/img812/4879/nmrmetatrader.png
Original size screenshots:
http://img196.imageshack.us/img196/8089/nmrtradestation.png
http://img820.imageshack.us/img820/4879/nmrmetatrader.png
#property indicator_separate_window #property indicator_buffers 2 #property indicator_color1 Magenta #property indicator_color2 Silver #property indicator_level1 0 #property indicator_levelcolor Gray #property indicator_levelstyle 2 extern int NMR_period = 40; extern bool Show_SD = true; extern int SD_len = 30; extern double SD_up = 2; extern double SD_dn = 2; double NMR[]; double SD[]; int init() { string nmrname = "NMR(" + NMR_period + ")"; string sdname = "NMR_SD(" + SD_len + ")"; IndicatorShortName(nmrname); IndicatorBuffers(2); SetIndexBuffer(0, NMR); SetIndexLabel(0, nmrname); SetIndexBuffer(1, SD); SetIndexLabel(1, sdname); return(0); } int start() { int limit, i, ii, counted_bars = IndicatorCounted(); if(Bars <= NMR_period) return(0); if(counted_bars < 0) counted_bars = 0; if(counted_bars > NMR_period) limit = Bars - counted_bars; else limit = Bars - NMR_period - 1; for(i = limit; i >= 0; i--) { double nmr2 = 0; for(ii = 1; ii <= NMR_period; ii++) nmr2 += (MathLog(Close[i+ii-1]) - MathLog(Close[i+ii])) * (MathSqrt(ii) - MathSqrt(ii-1)); NMR[i] = nmr2 * 1000; } if(Show_SD) for(i = limit; i >= 0; i--) if(i < Bars - NMR_period - 1 - SD_len) { if(NMR[i] == 0) SD[i] = 0; else if(NMR[i] > 0) SD[i] = iBandsOnArray(NMR, 0, SD_len, SD_up, 0, MODE_UPPER, i); else if(NMR[i] < 0) SD[i] = iBandsOnArray(NMR, 0, SD_len, SD_dn, 0, MODE_LOWER, i); } return(0); }
The source code is also available for download here:
-
NMM for MT4
Now NMM itself.
NMM is basically an average of Ocean Indexes with lookback from 1 to n, where n is NMM_period (40 by default).
So for example NMM(5) would be:
( OceanIndex(1) + OceanIndex(2) + OceanIndex(3) + OceanIndex(4) + OceanIndex(5) ) / 5
Original NMM on TradeStation:
http://img835.imageshack.us/img835/8959/nmmtradestation.png
NMM on Metatrader:
http://img820.imageshack.us/img820/5751/nmmmetatrader.png
Original size screenshots:
http://img833.imageshack.us/img833/8959/nmmtradestation.png
http://img20.imageshack.us/img20/5751/nmmmetatrader.png
#property indicator_separate_window #property indicator_buffers 2 #property indicator_color1 Magenta #property indicator_color2 Silver #property indicator_level1 0 #property indicator_levelcolor Gray #property indicator_levelstyle 2 extern int NMM_period = 40; extern bool Show_SD = true; extern int SD_len = 30; extern double SD_up = 2; extern double SD_dn = 2; double NMM[]; double SD[]; int init() { string nmmname = "NMM(" + NMM_period + ")"; string sdname = "NMM_SD(" + SD_len + ")"; IndicatorShortName(nmmname); IndicatorBuffers(2); SetIndexBuffer(0, NMM); SetIndexLabel(0, nmmname); SetIndexBuffer(1, SD); SetIndexLabel(1, sdname); return(0); } int start() { int limit, i, ii, counted_bars = IndicatorCounted(); if(Bars <= NMM_period) return(0); if(counted_bars < 0) counted_bars = 0; if(counted_bars > NMM_period) limit = Bars - counted_bars; else limit = Bars - NMM_period - 1; for(i = limit; i >= 0; i--) { double nmm2 = 0; for(ii = 1; ii <= NMM_period; ii++) nmm2 += (MathLog(Close[i]) - MathLog(Close[i+ii])) / MathSqrt(ii); NMM[i] = (nmm2 / NMM_period) * 1000; } if(Show_SD) for(i = limit; i >= 0; i--) if(i < Bars - NMM_period - 1 - SD_len) { if(NMM[i] == 0) SD[i] = 0; else if(NMM[i] > 0) SD[i] = iBandsOnArray(NMM, 0, SD_len, SD_up, 0, MODE_UPPER, i); else if(NMM[i] < 0) SD[i] = iBandsOnArray(NMM, 0, SD_len, SD_dn, 0, MODE_LOWER, i); } return(0); }
The source code is also available for download here:
For now I am showing the Ocean indis on the same chart for reference.
-
NMM Ocean Index for MT4
Next one is the NMM Ocean Index.
A slight discrepancy (i.e. a missing SD band's zero cross from below on 3/29) is due to the differences in price feeds, the math is correct.
Original NMM Ocean Index on TradeStation:
http://img195.imageshack.us/img195/7533/oceanindextradestation.png
NMM Ocean Index on Metatrader:
http://img853.imageshack.us/img853/438/oceanindexmetatrader.png
Original size screenshots:
http://img651.imageshack.us/img651/7533/oceanindextradestation.png
http://img40.imageshack.us/img40/438/oceanindexmetatrader.png
#property indicator_separate_window #property indicator_buffers 2 #property indicator_color1 Magenta #property indicator_color2 Silver #property indicator_level1 0 #property indicator_levelcolor Gray #property indicator_levelstyle 2 extern int NMM_LB = 21; extern bool Show_SD = true; extern int SD_len = 30; extern double SD_up = 2; extern double SD_dn = 2; double NMM[]; double SD[]; int init() { string nmmname = "NMM_Ocean_Index(" + NMM_LB + ")"; string sdname = "NMM_Ocean_Index_SD(" + SD_len + ")"; IndicatorShortName(nmmname); IndicatorBuffers(2); SetIndexBuffer(0, NMM); SetIndexLabel(0, nmmname); SetIndexBuffer(1, SD); SetIndexLabel(1, sdname); return(0); } int start() { int limit, i, counted_bars = IndicatorCounted(); if(Bars <= NMM_LB) return(0); if(counted_bars < 0) counted_bars = 0; if(counted_bars > NMM_LB) limit = Bars - counted_bars; else limit = Bars - NMM_LB - 1; for(i = limit; i >= 0; i--) NMM[i] = (MathLog(Close[i]) - MathLog(Close[i+NMM_LB])) / MathSqrt(NMM_LB) * 1000; if(Show_SD) for(i = limit; i >= 0; i--) if(i < Bars - NMM_LB - 1 - SD_len) { if(NMM[i] == 0) SD[i] = 0; else if(NMM[i] > 0) SD[i] = iBandsOnArray(NMM, 0, SD_len, SD_up, 0, MODE_UPPER, i); else if(NMM[i] < 0) SD[i] = iBandsOnArray(NMM, 0, SD_len, SD_dn, 0, MODE_LOWER, i); } return(0); }
The source code is also available for download here:
I take it chronologically as Pat is explaining them in the videos, so the next one will probably be NMR. These are the building blocks for the more advanced Ocean indis so even though these may not be the most useful directly I would have to program them anyway.
tgt123: I will get to those as well.
Grain Trader: not sure which ones you mean. In some of earlier posts I described what Ocean indis are in the TradeStation package I shared here.
-
NMA for MT4
Well, since no one seems to either have access to the advanced elite section of the TSD forum, or isn't willing to share, I started reprogramming Ocean Theory indicators from TradeStation to Metatrader myself, first one is NMA.
I may do a couple more and that's it because the TradeStation code is annoying.
Original NMA on TradeStation:
http://img404.imageshack.us/img404/3062/nmatradestation.png
NMA on Metatrader:
http://img69.imageshack.us/img69/2483/nmametatrader.png
Original size screenshots:
http://img521.imageshack.us/img521/3062/nmatradestation.png
http://img19.imageshack.us/img19/2483/nmametatrader.png
For some reason I can't post an attachment so I just paste the code here:
#property indicator_chart_window #property indicator_buffers 3 #property indicator_color1 Yellow #property indicator_color2 Gray #property indicator_color3 Gray extern int NMA_period = 40; extern bool Show_SD = true; extern int SD_len = 30; extern double SD_up = 2; extern double SD_dn = 2; double NMA[]; double SDup[]; double SDdn[]; int init() { string nmaname = "NMA(" + NMA_period + ")"; string sdname = "NMA_SD(" + SD_len + ")"; IndicatorShortName(nmaname); IndicatorBuffers(3); SetIndexBuffer(0, NMA); SetIndexLabel(0, nmaname); SetIndexBuffer(1, SDup); SetIndexLabel(1, sdname+"_up"); SetIndexBuffer(2, SDdn); SetIndexLabel(2, sdname+"_dn"); return(0); } int start() { int limit, i, ii, counted_bars = IndicatorCounted(); if(Bars <= MathMax(NMA_period, SD_len)) return(0); if(counted_bars < 0) counted_bars = 0; if(counted_bars > MathMax(NMA_period, SD_len)) limit = Bars - counted_bars; else limit = Bars - MathMax(NMA_period, SD_len) - 1; for(i = limit; i >= 0; i--) { double sum, abssum, ratio; sum = (MathLog(Close[i]) - MathLog(Close[i+1])) + (MathLog(Close[i+1]) - MathLog(Close[i+2])) * (MathSqrt(2)-1); for(ii = 2; ii < NMA_period; ii++) sum += (MathLog(Close[i+ii]) - MathLog(Close[i+ii+1])) * (MathSqrt(ii+1) - MathSqrt(ii)); abssum = MathAbs(sum); sum = 0; for(ii = 0; ii < NMA_period; ii++) sum += MathAbs(MathLog(Close[i+ii]) - MathLog(Close[i+ii+1])); if(sum != 0) ratio = abssum / sum; NMA[i] = NMA[i+1] + (Close[i] - NMA[i+1]) * ratio; if(Show_SD) { SDup[i] = NMA[i] + SD_up * iStdDev(Symbol(), Period(), SD_len, 0, 0, PRICE_CLOSE, i); SDdn[i] = NMA[i] - SD_dn * iStdDev(Symbol(), Period(), SD_len, 0, 0, PRICE_CLOSE, i); } } return(0); }
The source code is also available for download here:
Please suggest which Ocean indi would be the most useful to reprogram to Metatrader.
-
SpacyTrader: what you are saying is that governments are fake, they are just puppets controlled by the people behind the curtain who actually hold the real power. That corresponds with the "conspiracy theory" I stated and therefore I said: if that's really the case then they should simply cancel all those puppet governments right away and rule directly. It's just pointless to have fake governments, it serves no good to anyone, not even to those puppetmasters who are really in charge. There is no reason why they shouldn't rule directly. I am not happy about it but it would be simply better if we all knew what's really the reality.
You see, we are paying taxes to fake powerless governments. Doesn't it bother you? If I must pay taxes to anyone, instead of supporting fakes I would rather pay it to the real rulers. I would feel better, at least I would know who to hate. I am not saying they should come forward and say their names and show their faces, they could stay behind some nickname or whatever, but they should simply remove the fake powerless middlemen. You see my point? The current situation is just s t u p i d . If we have to live in the corporatocracy then let's be it, do it, say it, make it clear and get it over with. What is now is just schizophrenic pointless theater.
-
-
Would anyone with access to the TSD advanced elite forum be so kind and download all the indis from this thread?
http://www.forex-tsd.com/advanced-elite/22946-ocean-theory-based-indicators.html
For more details please see:
http://indo-investasi.com/showthread.php/17702-%28REQ%29-Ocean-Theory-indis-from-TSD-elite
Thanks a lot.
-
Upload is finished, I posted all the links, but apparently the post is waiting to be approved by the moderator (too long post or too many links?) so you just need to wait a bit.
In the meantime, before the post gets approved, I uploaded the vids for direct watching also here:
DVD1-DVD9
Bonus DVD
- Grain Trader, cho_7288, C0UNDE and 3 others
-
6
-
I just finished all uploading, so I will put all links into this single post.
Introduction ebook:
http://uloz.to/xFyoPQG/m-sloman-jim-ocean-theory-an-introduction-pdf
Whole package except the videos:
http://uloz.to/x83ecYT/jim-sloman-ocean-theory-7z
All videos:
http://uloz.to/xFhAnF2/ocean-theory-dvd1-avi
http://uloz.to/xoNqUFA/ocean-theory-dvd2-avi
http://uloz.to/xpm1rYT/ocean-theory-dvd3-avi
http://uloz.to/x1DJPqJ/ocean-theory-dvd4-avi
http://uloz.to/xY9E2t8/ocean-theory-dvd5-avi
http://uloz.to/xt4bRVE/ocean-theory-dvd6-avi
http://uloz.to/xtUBifX/ocean-theory-dvd7-avi
http://uloz.to/xHVs49u/ocean-theory-dvd8-avi
http://uloz.to/xP1aG9K/ocean-theory-dvd9-avi
http://uloz.to/xkE42VW/ocean-theory-bonus-dvd-avi
This is the package I originally downloaded (for the reference):
http://uloz.to/xLUwUFA/jim-sloman-ocean-theory-7z-001
http://uloz.to/xMv8nGd/jim-sloman-ocean-theory-7z-002
http://uloz.to/xhHnmcv/jim-sloman-ocean-theory-7z-003
This is VMware image with preinstalled TradeStation + live forex feed + Ocean Theory indicators:
http://uloz.to/xAPJSAD/tradestationoceantheory-7z
All uloz.to uploads:
http://www.ulozto.net/hledej/?q=ocean+theory
I also set the videos to be available for direct viewing at uloz.to Live, so when they get indexed they will hopefully be accessible here:
http://www.ulozto.net/hledej/?service=live&q=ocean+theory
And here are screenshots of all 8 pages of the Ocean Theory MT4/MT5 thread at TSD advanced elite forum:
http://img684.imageshack.us/img684/3113/oceantheorytsdadvancede.png
http://img14.imageshack.us/img14/3113/oceantheorytsdadvancede.png
http://img560.imageshack.us/img560/3113/oceantheorytsdadvancede.png
http://img7.imageshack.us/img7/3113/oceantheorytsdadvancede.png
http://img593.imageshack.us/img593/3113/oceantheorytsdadvancede.png
http://img685.imageshack.us/img685/3113/oceantheorytsdadvancede.png
http://img14.imageshack.us/img14/3855/oceantheorytsdadvancedez.png
http://img818.imageshack.us/img818/3113/oceantheorytsdadvancede.png
Now we just need a good soul with access to the advanced elite TSD forum who would be willing to download and share the files.
Instructions for VMware image: before you run the image in VMware, set the memory (1 GB RAM is enough, probably even 512 MB would be sufficient) and the number of CPU cores. Then start it and when Windows XP boots run Forexite QuoteRoom via the desktop shortcut. That would start live Forex feed and also download all the history data since the last run and import it into the Omega Research's GlobalServer (live feed source for the TradeStation) which is launched by QuoteRoom. This all is done automatically, you just need to start QuoteRoom. Then start the TradeStation and select "Work Offline". This will open the TradeStation with access to the history data as well as to the live feed via the "Forexite broker->QuoteRoom->GlobalServer->TradeStation" chain. Currently there is a history data from 01/02/2011 to 03/30/2012 imported into the GlobalServer, you can import more of course.
This whole VMware thing is just to have a possibility to play a bit with Ocean Theory indicators until we get the Metatrader versions.
-
Here are the screenshots of the mladen's Ocean Theory thread at the TSD advanced elite section:
-
Well, my remark was particularly focused on the governments. Banks may have expected it, sure. Big got bigger, rich got richer. But did actually the governments expect it? Because I can't see what good this situation has done to any government. All governments across the globe lost votes, they are blamed by the citizens for letting it to happen. So I see only two possible options: either governments really didn't expect it which means they are just s t u p i d and incompetent. Or they did expect it but believed they could prevent it. Which just again means they are s t u p i d and incompetent.
I know it's no news that governments are s t u p i d , but this was quite a very big and serious issue with the impact on millions of people and they failed big time. Now just imagine if the subject wasn't the finance but, say, a nuclear war. With the same ease they could just wipe the whole world tomorrow because of their s t u p i d i t y and that honestly scares me. Doesn't it you?
<conspiracy_theory>
Only possible partial excuse would be that the governments were victims of a conspiracy plot aiming at destroying all governments and bringing "the new world order". Well, if that is the case then they may just do it right away and get it over with. At least we would know what's up and who is who. Having incapable governments is just pointless. And while being at it they could as well cancel all borders, all import/export and immigration/emigration regulations, keep just a single world-wide currency and dissolve all financial markets. None of that ever served any good to the humanity anyway.
</conspiracy_theory>
Ok, I will finish the uploading in about 5 hours. I will publish the original 2.12 GB package as well as all 10 videos each separately. Also the 1.1 GB vmware package with TradeStation's Ocean indis installed.
-
Unfortunately I don't remember the link, but the thread was created by mladen, it was probably in 2009 or 2010 and he was posting all Ocean indicators (NMA, NMC, NMM etc.) in this very same thread one by one (probably as he was programming it) and he always quoted rather long text description of a indi and then he put the .mq4 as the attachment. There were no screenshots (or at least they were not visible while being on trial subscription).
It is in the advanced elite section. I think if you search advanced elite for "ocean" you should find it right away, even just by listing manually, there are just a few pages there if I remember correctly.
I signed for advanced elite trial there a week or two ago just to check it out but since there were no screenshots paying $89 seemed a bit too high price for buying something I couldn't even see in advance what it looks like and whether it's really the same algorithms as TradeStation's original. Especially because mladen mentioned that he was looking for a Ocean Theory manual or videos or something like that (or at least I think I noticed such his remark, if I am not mistaken) and this was after he posted all those indis, so I got an impression that he maybe might not have been totally sure whether his implementations are totally faithful to the original algorithms.
If you were having difficulties in finding this thread I can probably sign for a advanced elite trial there again, to show you.
Just wait till I upload all the videos from the seminar, Pat Raffalovich is step by step presenting all these indis, as building stones, and it was (at least for me) quite thrilling, I was watching first several hours with eyes on the screen as watching a good movie :-) Then it gets a bit more complicated and without the manual it would be too difficult to follow all that. The free youtube videos are also helpful. Then the bonus video with Jim Sloman precisely describing all this credit, mortgage and reality estate bubbles, injecting more and more money into the system by the fed, rapidly rising inflation, growth of toxic loans and other aspects, and how it all is inevitably going to burst soon turning into the big global economic depression, that was truly just jaw dropping. It makes you think how is it that some guy (sure he is very talented and everything, but still he is just "some guy") knew it all in 2005, several years before it actually happened, and neither banks nor the governments knew it so they could have prevented it. It's just unbelievable, and sad.
- Grain Trader and nilvano
-
2
-
Of course, I just had to upload it.
This 56.9 MB package contains everything as the original 2.12 GB package except the videos:
http://uloz.to/x83ecYT/jim-sloman-ocean-theory-7z
I wish anyone with access to TSD Elite would be so kind and download and share the Ocean indis from there..
-
-
I originally started this thread in hope that someone with access to the advanced elite section of TSD forum would be willing to download and share Ocean Theory indicators reprogrammed there by mladen to MT4 platform. However no one was found so I ended up programming those indicators myself.
09-28-2012 UPDATE
New bunch of mirrors (the latest versions of my implementation of the Ocean indicators + Ocean indicators from TSD elite):
08-26-2012 UPDATE
Another variant indi - Ocn_FastNMAvsNMA_MTF (MTF version of Ocn_FastNMAvsNMA):
08-21-2012 UPDATE
Two new (or rather variations of existing) indis Ocn_FastNMAvsNMA 1.01 and Ocn_FastNMAvsNMA_NMS 1.00:
08-15-2012 UPDATE
At last, though a little late in the day, the indicators reprogrammed by mladen from TSD forum have been contributed by human18:
A few mirrors:
07-28-2012 UPDATE
Original Ocean Theory files for the Tradestation, including all the DVDs, uploaded to some 20 mirrors:
07-24-2012 UPDATE
The latest version:
05-07-2012 UPDATE
Ocn_BTXx version 1.21:
05-06-2012 UPDATE
BTX_2line for MT4:
05-01-2012 UPDATE
BTX for MT4 added:
04-15-2012 UPDATE
The latest version:
04-12-2012 UPDATE
The download link with the latest versions of all remade indicators so far:
The download links with videos, manuals and indicators for TradeStation:
My original message:
----------------------------------------------------------------
Hi everyone,
does anyone happen to have Ocean Theory indis from the TSD elite section? Or perhaps could someone please download it from there and share here? There is a whole dedicated Ocean Theory thread on the TSD forum, created by mladen. He reprogrammed probably all those indicators to MT4 platform.
I know there are already a few topics with TSD elite indis here on this forum, but I just can't find any Ocean indi in any downloadable file posted. Or I am just blind (am I?). The names of the most important Ocean indis are: NDX, NST, NXC, NMA, NMC, NMM, NMR, NMS.
These are not just another ordinary indicators. The math behind was created by Jim Sloman, that guy is for real, just watch some of his vids (links bellow). He has incredible knowledge and insight.
I was fortunate to download a 2.12 GB package (the source link is already dead), it contains:
- some pdf files: 213 pages Ocean indis manual, 53 pages about strategy (but this is related to only a few Ocean indis), 90 pages Ocean Theory introduction book
- Ocean indis for TradeStation (including the source codes)
- 10 DVD videos
Nine of those videos are the recordings from the 3-days Ocean Theory seminar, presented by Pat Raffalovich who co-created the Ocean Theory with Jim and programmed it all. These videos are very valuable because he step by step explains each indicator and its math and how to use it. Without it (and the books) the indis would be very difficult to use, if at all.
The last video is a bonus with Jim solo speaking for 49 minutes about his views on the current state and the future of global finance. In this video he very precisely described, explained and predicted the 2008-2010 financial crysis. What is so amazing about that? Well, the recording is from Feb 26, 2005.
My home upload speed is terrible, but I will try to find a way how to reupload this whole package, it's really worth it.
Also, I have adapted a VMware image with fully working TradeStation 8.7 including live Forex feed (with history data as well), which I downloaded somewhere off the Internet, and installed there all Ocean Theory indis. I will try to upload this package too (several GB).
I am not saying this is a holy grail or anything, it's actually quite complicated to learn it all, but of all those tens and hundreds books, trading systems and indicators, its main focus on non-arbitrariness and meaningful math makes it just a really worth a try.
So please, if anyone have already downloaded, or could download, these Ocean Theory indicators from TSD elite, please please share it.
A few links:
http://www.manyblessings.net/oceantheory.html
- hellboy1713006415, taipan, for-ex and 9 others
-
12
- some pdf files: 213 pages Ocean indis manual, 53 pages about strategy (but this is related to only a few Ocean indis), 90 pages Ocean Theory introduction book
Ocean Theory indis from TSD elite
in MetaTrader Indicators
Posted
Fast NMA for MT4
Fast NMA.
On the screenshots the regular NMA is yellow/gray dotted line, fast NMA is light/dark blue solid line. This one seems to me quite very useful for short-term support/resistance.
Original Fast NMA on TradeStation:
http://img210.imageshack.us/img210/4584/fastnmatradestation.png
Fast NMA on Metatrader:
http://img829.imageshack.us/img829/2144/fastnmametatrader.png
Original size screenshots:
http://img821.imageshack.us/img821/4584/fastnmatradestation.png
http://img3.imageshack.us/img3/2144/fastnmametatrader.png
The source code is also available for download here:
http://pastebin.com/1m9EavNT