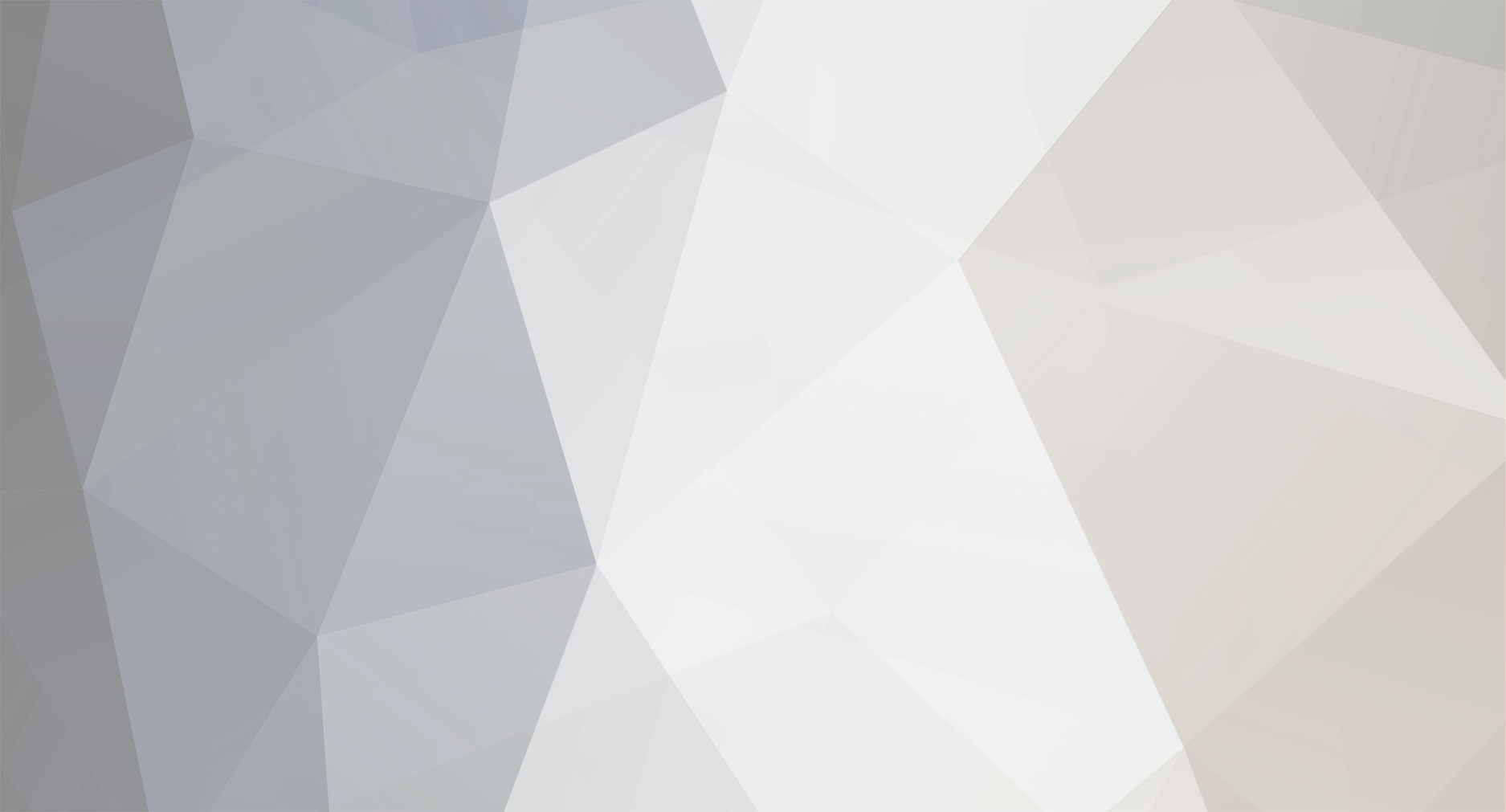
udc
-
Posts
224 -
Joined
-
Last visited
-
Days Won
24
Content Type
Profiles
Forums
Articles
Posts posted by udc
-
-
Ocn_NMSx for MT4
Â
Ocn_NMSx.
Â
Â
#property indicator_separate_window #property indicator_buffers 6 #property indicator_color1 Magenta #property indicator_color2 Lime #property indicator_color3 Silver #property indicator_color4 Silver #property indicator_color5 Yellow #property indicator_color6 Aqua #property indicator_level1 0 #property indicator_levelcolor Gray #property indicator_levelstyle 2 extern int NMS_period = 40; extern bool Show_ZH = true; extern double ZH_displacement = 1; extern int ZH_symbol = 115; extern bool Show_SD = true; extern int SD_len = 30; extern double SD_up = 2; extern double SD_dn = 2; extern bool Show_SD_1line_only = true; extern bool Show_SD_2lines_up = true; extern bool Show_SD_2lines_dn = true; extern int NMA_period = 40; extern int NMA_LB_min = 8; extern bool Show_NMA = true; extern bool Show_FastNMA = true; double NMS[]; double ZH[]; double SD[]; double SD2[]; double NMS_NMA[]; double NMS_FastNMA[]; int init() { string nmsname = "NMS(" + NMS_period + ")"; IndicatorShortName(nmsname); IndicatorBuffers(6); SetIndexBuffer(0, NMS); SetIndexLabel(0, nmsname); SetIndexBuffer(1, ZH); SetIndexLabel(1, "NMS_ZH"); SetIndexStyle(1, DRAW_ARROW); SetIndexArrow(1, ZH_symbol); SetIndexBuffer(2, SD); SetIndexBuffer(3, SD2); if(Show_SD_1line_only) { SetIndexLabel(2, "NMS_SD(" + SD_len + ")"); SetIndexLabel(3, "unused"); } else { if(Show_SD_2lines_up) SetIndexLabel(2, "NMS_SD_up(" + SD_len + ")"); else SetIndexLabel(2, "unused"); if(Show_SD_2lines_dn) SetIndexLabel(3, "NMS_SD_dn(" + SD_len + ")"); else SetIndexLabel(3, "unused"); } SetIndexBuffer(4, NMS_NMA); SetIndexBuffer(5, NMS_FastNMA); if(Show_NMA) SetIndexLabel(4, "NMS_NMA(" + NMA_period + ")"); else SetIndexLabel(4, "unused"); if(Show_FastNMA) SetIndexLabel(5, "NMS_FastNMA(" + NMA_period + ", " + NMA_LB_min + ")"); else SetIndexLabel(5, "unused"); return(0); } int start() { int limit, i, ii, counted_bars = IndicatorCounted(); double nms2, sum, abssum, ratio, nmsnum, maxnms; if(Bars <= NMS_period) return(0); if(counted_bars < 0) counted_bars = 0; if(counted_bars > NMS_period) limit = Bars - counted_bars; else limit = Bars - NMS_period - 1; for(i = limit; i >= 0; i--) { nms2 = 0; for(ii = 1; ii <= NMS_period; ii++) { double oLRSlope = 0, SumXY = 0, SumY = 0, SumX, SumXSqr, Divisor; if(ii > 1) { SumX = ii * (ii - 1) * 0.5; SumXSqr = ii * (ii - 1) * (2 * ii - 1) * 1/6; Divisor = (SumX * SumX) - ii * SumXSqr; for(int iii = 0; iii < ii; iii++) { SumXY += iii * MathLog(Close[i+iii]); SumY += MathLog(Close[i+iii]); } oLRSlope = (ii * SumXY - SumX * SumY) / Divisor; } nms2 += oLRSlope * MathSqrt(ii); } NMS[i] = nms2 * 100; if(Show_ZH) { if(NMS[i] == 0) ZH[i] = ZH_displacement; else ZH[i] = EMPTY_VALUE; } } if(Show_SD) for(i = limit; i >= 0; i--) if(i < Bars - NMS_period - 1 - SD_len) { if(Show_SD_1line_only) { if(NMS[i] == 0) SD[i] = 0; else if(NMS[i] > 0) SD[i] = iBandsOnArray(NMS, 0, SD_len, SD_up, 0, MODE_UPPER, i); else if(NMS[i] < 0) SD[i] = iBandsOnArray(NMS, 0, SD_len, SD_dn, 0, MODE_LOWER, i); } else { if(NMS[i] == 0) { if(Show_SD_2lines_up) SD[i] = 0; if(Show_SD_2lines_dn) SD2[i] = 0; } else { if(Show_SD_2lines_up) SD[i] = iBandsOnArray(NMS, 0, SD_len, SD_up, 0, MODE_UPPER, i); if(Show_SD_2lines_dn) SD2[i] = iBandsOnArray(NMS, 0, SD_len, SD_dn, 0, MODE_LOWER, i); } } } if(Show_NMA) for(i = limit; i >= 0; i--) if(i < Bars - NMS_period - 1 - NMA_period) { ratio = 0; sum = (NMS[i] - NMS[i+1]) + (NMS[i+1] - NMS[i+2]) * (MathSqrt(2)-1); for(ii = 2; ii < NMA_period; ii++) sum += (NMS[i+ii] - NMS[i+ii+1]) * (MathSqrt(ii+1) - MathSqrt(ii)); abssum = MathAbs(sum); sum = 0; for(ii = 0; ii < NMA_period; ii++) sum += MathAbs(NMS[i+ii] - NMS[i+ii+1]); if(sum != 0) ratio = abssum / sum; NMS_NMA[i] = NMS_NMA[i+1] + (NMS[i] - NMS_NMA[i+1]) * ratio; } if(Show_FastNMA) for(i = limit; i >= 0; i--) if(i < Bars - NMS_period - 1 - NMA_period) { maxnms = 0; ratio = 0; int NMA_LB_max; for(ii = 1; ii <= NMA_period; ii++) { nmsnum = (NMS[i] - NMS[i+ii]) / MathSqrt(ii); if(MathAbs(nmsnum) > maxnms) { maxnms = MathAbs(nmsnum); NMA_LB_max = ii; } } if(NMA_LB_max < NMA_LB_min) NMA_LB_max = NMA_LB_min; sum = (NMS[i] - NMS[i+1]) + (NMS[i+1] - NMS[i+2]) * (MathSqrt(2)-1); for(ii = 2; ii < NMA_LB_max; ii++) sum += (NMS[i+ii] - NMS[i+ii+1]) * (MathSqrt(ii+1) - MathSqrt(ii)); abssum = MathAbs(sum); sum = 0; for(ii = 0; ii < NMA_LB_max; ii++) sum += MathAbs(NMS[i+ii] - NMS[i+ii+1]); if(sum != 0) ratio = abssum / sum; NMS_FastNMA[i] = NMS_FastNMA[i+1] + (NMS[i] - NMS_FastNMA[i+1]) * ratio; } return(0); }
Â
The source code is also available for download here:
- C0UNDE, damo1713005996, mashki and 4 others
-
7
-
Ocn_NMCx for MT4
Â
Similarly as Ocn_NMC2x, here is Ocn_NMCx.
Â
Â
#property indicator_separate_window #property indicator_buffers 6 #property indicator_color1 Magenta #property indicator_color2 Lime #property indicator_color3 Silver #property indicator_color4 Silver #property indicator_color5 Yellow #property indicator_color6 Aqua #property indicator_level1 0 #property indicator_levelcolor Gray #property indicator_levelstyle 2 extern int NMC_period = 40; extern bool Show_ZH = true; extern double ZH_displacement = 1; extern int ZH_symbol = 115; extern bool Show_SD = true; extern int SD_len = 30; extern double SD_up = 2; extern double SD_dn = 2; extern bool Show_SD_1line_only = true; extern bool Show_SD_2lines_up = true; extern bool Show_SD_2lines_dn = true; extern int NMA_period = 40; extern int NMA_LB_min = 8; extern bool Show_NMA = true; extern bool Show_FastNMA = true; double NMC[]; double ZH[]; double SD[]; double SD2[]; double NMC_NMA[]; double NMC_FastNMA[]; int init() { string nmcname = "NMC(" + NMC_period + ")"; IndicatorShortName(nmcname); IndicatorBuffers(6); SetIndexBuffer(0, NMC); SetIndexLabel(0, nmcname); SetIndexBuffer(1, ZH); SetIndexLabel(1, "NMC_ZH"); SetIndexStyle(1, DRAW_ARROW); SetIndexArrow(1, ZH_symbol); SetIndexBuffer(2, SD); SetIndexBuffer(3, SD2); if(Show_SD_1line_only) { SetIndexLabel(2, "NMC_SD(" + SD_len + ")"); SetIndexLabel(3, "unused"); } else { if(Show_SD_2lines_up) SetIndexLabel(2, "NMC_SD_up(" + SD_len + ")"); else SetIndexLabel(2, "unused"); if(Show_SD_2lines_dn) SetIndexLabel(3, "NMC_SD_dn(" + SD_len + ")"); else SetIndexLabel(3, "unused"); } SetIndexBuffer(4, NMC_NMA); SetIndexBuffer(5, NMC_FastNMA); if(Show_NMA) SetIndexLabel(4, "NMC_NMA(" + NMA_period + ")"); else SetIndexLabel(4, "unused"); if(Show_FastNMA) SetIndexLabel(5, "NMC_FastNMA(" + NMA_period + ", " + NMA_LB_min + ")"); else SetIndexLabel(5, "unused"); return(0); } int start() { int limit, i, ii, counted_bars = IndicatorCounted(); double nmm, nmr, nmm2, nmr2, avg, sign, sum, abssum, ratio, nmcnum, maxnmc; if(Bars <= NMC_period) return(0); if(counted_bars < 0) counted_bars = 0; if(counted_bars > NMC_period) limit = Bars - counted_bars; else limit = Bars - NMC_period - 1; for(i = limit; i >= 0; i--) { nmm2 = 0; nmr2 = 0; for(ii = 1; ii <= NMC_period; ii++) { nmm2 += (MathLog(Close[i]) - MathLog(Close[i+ii])) / MathSqrt(ii); nmr2 += (MathLog(Close[i+ii-1]) - MathLog(Close[i+ii])) * (MathSqrt(ii) - MathSqrt(ii-1)); } nmm = (nmm2 / NMC_period) * 1000; nmr = nmr2 * 1000; avg = ((MathAbs(nmm) * nmr) + (MathAbs(nmr) * nmm)) / 2; if(avg > 0) sign = 1; else if(avg < 0) sign = -1; else sign = 0; NMC[i] = sign * MathSqrt(MathAbs(avg)); if(Show_ZH) { if(NMC[i] == 0) ZH[i] = ZH_displacement; else ZH[i] = EMPTY_VALUE; } } if(Show_SD) for(i = limit; i >= 0; i--) if(i < Bars - NMC_period - 1 - SD_len) { if(Show_SD_1line_only) { if(NMC[i] == 0) SD[i] = 0; else if(NMC[i] > 0) SD[i] = iBandsOnArray(NMC, 0, SD_len, SD_up, 0, MODE_UPPER, i); else if(NMC[i] < 0) SD[i] = iBandsOnArray(NMC, 0, SD_len, SD_dn, 0, MODE_LOWER, i); } else { if(NMC[i] == 0) { if(Show_SD_2lines_up) SD[i] = 0; if(Show_SD_2lines_dn) SD2[i] = 0; } else { if(Show_SD_2lines_up) SD[i] = iBandsOnArray(NMC, 0, SD_len, SD_up, 0, MODE_UPPER, i); if(Show_SD_2lines_dn) SD2[i] = iBandsOnArray(NMC, 0, SD_len, SD_dn, 0, MODE_LOWER, i); } } } if(Show_NMA) for(i = limit; i >= 0; i--) if(i < Bars - NMC_period - 1 - NMA_period) { ratio = 0; sum = (NMC[i] - NMC[i+1]) + (NMC[i+1] - NMC[i+2]) * (MathSqrt(2)-1); for(ii = 2; ii < NMA_period; ii++) sum += (NMC[i+ii] - NMC[i+ii+1]) * (MathSqrt(ii+1) - MathSqrt(ii)); abssum = MathAbs(sum); sum = 0; for(ii = 0; ii < NMA_period; ii++) sum += MathAbs(NMC[i+ii] - NMC[i+ii+1]); if(sum != 0) ratio = abssum / sum; NMC_NMA[i] = NMC_NMA[i+1] + (NMC[i] - NMC_NMA[i+1]) * ratio; } if(Show_FastNMA) for(i = limit; i >= 0; i--) if(i < Bars - NMC_period - 1 - NMA_period) { maxnmc = 0; ratio = 0; int NMA_LB_max; for(ii = 1; ii <= NMA_period; ii++) { nmcnum = (NMC[i] - NMC[i+ii]) / MathSqrt(ii); if(MathAbs(nmcnum) > maxnmc) { maxnmc = MathAbs(nmcnum); NMA_LB_max = ii; } } if(NMA_LB_max < NMA_LB_min) NMA_LB_max = NMA_LB_min; sum = (NMC[i] - NMC[i+1]) + (NMC[i+1] - NMC[i+2]) * (MathSqrt(2)-1); for(ii = 2; ii < NMA_LB_max; ii++) sum += (NMC[i+ii] - NMC[i+ii+1]) * (MathSqrt(ii+1) - MathSqrt(ii)); abssum = MathAbs(sum); sum = 0; for(ii = 0; ii < NMA_LB_max; ii++) sum += MathAbs(NMC[i+ii] - NMC[i+ii+1]); if(sum != 0) ratio = abssum / sum; NMC_FastNMA[i] = NMC_FastNMA[i+1] + (NMC[i] - NMC_FastNMA[i+1]) * ratio; } return(0); }
Â
The source code is also available for download here:
-
Ocn_NMC2x for MT4
Â
Ok, so here is a prototype of an NMC2 indicator with all functions incorporated, plus some new features.
Â
http://img801.imageshack.us/img801/5536/ocnnmc2x.png
Â
First, there is a new feature showing the zero hits using a specified symbol. You can select the symbol from the list:
http://img855.imageshack.us/img855/4386/mt4symbols.png
Â
Also, you can select the vertical displacement of this symbol. And of course you can turn it off.
Â
Second, there is an option to show either a single SD line (showing either upper or lower SD band based on the position of NMC2) as was so far, or you can choose to show both lower and upper SD bands or only one of them.
Showing both bands may be useful as can be seen in the screenshot on the right around 5 Apr 09:00.
Â
Lastly, you can selective turn on/off the NMA and FastNMA of NMC2.
Â
Â
Check it out and tell me whether you can think of any other feature or modification, before I remake all other Ocean indis in the same way.
From now on I will also prefix these final version with "Ocn_" string so it is sorted in the Metatrader all together.
Â
Original size screenshots:
http://img571.imageshack.us/img571/5536/ocnnmc2x.png
Â
#property indicator_separate_window #property indicator_buffers 6 #property indicator_color1 Magenta #property indicator_color2 Lime #property indicator_color3 Silver #property indicator_color4 Silver #property indicator_color5 Yellow #property indicator_color6 Aqua #property indicator_level1 0 #property indicator_levelcolor Gray #property indicator_levelstyle 2 extern int NMC2_period = 40; extern bool Use_NMR_instead_NMM = false; extern bool Show_ZH = true; extern double ZH_displacement = 1; extern int ZH_symbol = 115; extern bool Show_SD = true; extern int SD_len = 30; extern double SD_up = 2; extern double SD_dn = 2; extern bool Show_SD_1line_only = true; extern bool Show_SD_2lines_up = true; extern bool Show_SD_2lines_dn = true; extern int NMA_period = 40; extern int NMA_LB_min = 8; extern bool Show_NMA = true; extern bool Show_FastNMA = true; double NMC2[]; double ZH[]; double SD[]; double SD2[]; double NMC2_NMA[]; double NMC2_FastNMA[]; int init() { string whichone = "NMM"; if(Use_NMR_instead_NMM) whichone = "NMR"; string nmc2name = "NMC2(" + NMC2_period + ", using " + whichone + ")"; IndicatorShortName(nmc2name); IndicatorBuffers(6); SetIndexBuffer(0, NMC2); SetIndexLabel(0, nmc2name); SetIndexBuffer(1, ZH); SetIndexLabel(1, "NMC2_ZH"); SetIndexStyle(1, DRAW_ARROW); SetIndexArrow(1, ZH_symbol); SetIndexBuffer(2, SD); SetIndexBuffer(3, SD2); if(Show_SD_1line_only) { SetIndexLabel(2, "NMC2_SD(" + SD_len + ")"); SetIndexLabel(3, "unused"); } else { if(Show_SD_2lines_up) SetIndexLabel(2, "NMC2_SD_up(" + SD_len + ")"); else SetIndexLabel(2, "unused"); if(Show_SD_2lines_dn) SetIndexLabel(3, "NMC2_SD_dn(" + SD_len + ")"); else SetIndexLabel(3, "unused"); } SetIndexBuffer(4, NMC2_NMA); SetIndexBuffer(5, NMC2_FastNMA); if(Show_NMA) SetIndexLabel(4, "NMC2_NMA(" + NMA_period + ")"); else SetIndexLabel(4, "unused"); if(Show_FastNMA) SetIndexLabel(5, "NMC2_FastNMA(" + NMA_period + ", " + NMA_LB_min + ")"); else SetIndexLabel(5, "unused"); return(0); } int start() { int limit, i, ii, counted_bars = IndicatorCounted(); double nms, nmm, nmr, nms2, nmm2, nmr2, whichone, avg, sign, sum, abssum, ratio, nmc2num, maxnmc2; if(Bars <= NMC2_period) return(0); if(counted_bars < 0) counted_bars = 0; if(counted_bars > NMC2_period) limit = Bars - counted_bars; else limit = Bars - NMC2_period - 1; for(i = limit; i >= 0; i--) { nms2 = 0; nmm2 = 0; nmr2 = 0; for(ii = 1; ii <= NMC2_period; ii++) { double oLRSlope = 0, SumXY = 0, SumY = 0, SumX, SumXSqr, Divisor; if(ii > 1) { SumX = ii * (ii - 1) * 0.5; SumXSqr = ii * (ii - 1) * (2 * ii - 1) * 1/6; Divisor = (SumX * SumX) - ii * SumXSqr; for(int iii = 0; iii < ii; iii++) { SumXY += iii * MathLog(Close[i+iii]); SumY += MathLog(Close[i+iii]); } oLRSlope = (ii * SumXY - SumX * SumY) / Divisor; } nms2 += oLRSlope * MathSqrt(ii); nmm2 += (MathLog(Close[i]) - MathLog(Close[i+ii])) / MathSqrt(ii); nmr2 += (MathLog(Close[i+ii-1]) - MathLog(Close[i+ii])) * (MathSqrt(ii) - MathSqrt(ii-1)); } nms = nms2 * 100; nmm = (nmm2 / NMC2_period) * 1000; nmr = nmr2 * 1000; if(Use_NMR_instead_NMM) whichone = nmr; else whichone = nmm; avg = ((MathAbs(nms) * whichone) + (MathAbs(whichone) * nms)) / 2; if(avg > 0) sign = 1; else if(avg < 0) sign = -1; else sign = 0; NMC2[i] = sign * MathSqrt(MathAbs(avg)); if(Show_ZH) { if(NMC2[i] == 0) ZH[i] = ZH_displacement; else ZH[i] = EMPTY_VALUE; } } if(Show_SD) for(i = limit; i >= 0; i--) if(i < Bars - NMC2_period - 1 - SD_len) { if(Show_SD_1line_only) { if(NMC2[i] == 0) SD[i] = 0; else if(NMC2[i] > 0) SD[i] = iBandsOnArray(NMC2, 0, SD_len, SD_up, 0, MODE_UPPER, i); else if(NMC2[i] < 0) SD[i] = iBandsOnArray(NMC2, 0, SD_len, SD_dn, 0, MODE_LOWER, i); } else { if(NMC2[i] == 0) { if(Show_SD_2lines_up) SD[i] = 0; if(Show_SD_2lines_dn) SD2[i] = 0; } else { if(Show_SD_2lines_up) SD[i] = iBandsOnArray(NMC2, 0, SD_len, SD_up, 0, MODE_UPPER, i); if(Show_SD_2lines_dn) SD2[i] = iBandsOnArray(NMC2, 0, SD_len, SD_dn, 0, MODE_LOWER, i); } } } if(Show_NMA) for(i = limit; i >= 0; i--) if(i < Bars - NMC2_period - 1 - NMA_period) { ratio = 0; sum = (NMC2[i] - NMC2[i+1]) + (NMC2[i+1] - NMC2[i+2]) * (MathSqrt(2)-1); for(ii = 2; ii < NMA_period; ii++) sum += (NMC2[i+ii] - NMC2[i+ii+1]) * (MathSqrt(ii+1) - MathSqrt(ii)); abssum = MathAbs(sum); sum = 0; for(ii = 0; ii < NMA_period; ii++) sum += MathAbs(NMC2[i+ii] - NMC2[i+ii+1]); if(sum != 0) ratio = abssum / sum; NMC2_NMA[i] = NMC2_NMA[i+1] + (NMC2[i] - NMC2_NMA[i+1]) * ratio; } if(Show_FastNMA) for(i = limit; i >= 0; i--) if(i < Bars - NMC2_period - 1 - NMA_period) { maxnmc2 = 0; ratio = 0; int NMA_LB_max; for(ii = 1; ii <= NMA_period; ii++) { nmc2num = (NMC2[i] - NMC2[i+ii]) / MathSqrt(ii); if(MathAbs(nmc2num) > maxnmc2) { maxnmc2 = MathAbs(nmc2num); NMA_LB_max = ii; } } if(NMA_LB_max < NMA_LB_min) NMA_LB_max = NMA_LB_min; sum = (NMC2[i] - NMC2[i+1]) + (NMC2[i+1] - NMC2[i+2]) * (MathSqrt(2)-1); for(ii = 2; ii < NMA_LB_max; ii++) sum += (NMC2[i+ii] - NMC2[i+ii+1]) * (MathSqrt(ii+1) - MathSqrt(ii)); abssum = MathAbs(sum); sum = 0; for(ii = 0; ii < NMA_LB_max; ii++) sum += MathAbs(NMC2[i+ii] - NMC2[i+ii+1]); if(sum != 0) ratio = abssum / sum; NMC2_FastNMA[i] = NMC2_FastNMA[i+1] + (NMC2[i] - NMC2_FastNMA[i+1]) * ratio; } return(0); }
Â
The source code is also available for download here:
- mashki, damo1713005996, C0UNDE and 3 others
-
6
-
Thanks :)
Â
Ok, so what is left from the original Ocean pack is: NDX, NST and NXC. Then there are BTX and STX from the Ocean Plus, those have their logic moved to the external DLL, so I will see if the DLL will work in Metatrader.
Â
Also, I was thinking about remaking the so far done Ocean indis into a single version of each, i.e. a single NMA indi with NMA and FastNMA, a single NMC indi with MAs and SDs etc., with each line selectively switchable on/off. It wouldn't bring any new value, it would be just for convenience. Because I noticed on some youtube videos Jim is using for example NMC2 with OcnMAs as well as with SDs at the same time and on top of that there is not only one side of SD but both sides. So I was just thinking I may as well just make a single indi for each algorithm with all possible features built in all together, instead of having 2-3 or even more indi variants for each algo. What do you think, would it be worth the effort?
-
NMC2_with_Ocn_MAs for MT4
Â
NMC2_with_Ocn_MAs.
Â
Â
Original NMC2_with_Ocn_MAs on TradeStation:
http://img834.imageshack.us/img834/7189/nmc2withocnmastradestat.png
Â
NMC2_with_Ocn_MAs on Metatrader:
http://img31.imageshack.us/img31/6079/nmc2withocnmasmetatrade.png
Â
Original size screenshots:
http://img17.imageshack.us/img17/7189/nmc2withocnmastradestat.png
http://img534.imageshack.us/img534/6079/nmc2withocnmasmetatrade.png
Â
#property indicator_separate_window #property indicator_buffers 3 #property indicator_color1 Magenta #property indicator_color2 Yellow #property indicator_color3 Aqua #property indicator_level1 0 #property indicator_levelcolor Gray #property indicator_levelstyle 2 extern int NMC2_period = 40; extern bool Use_NMR_instead_NMM = false; extern int NMA_period = 40; extern int NMA_LB_min = 8; double NMC2[]; double NMC2_NMA[]; double NMC2_FastNMA[]; int init() { string whichone = "NMM"; if(Use_NMR_instead_NMM) whichone = "NMR"; IndicatorShortName("NMC2_with_Ocn_MAs(" + NMC2_period + ", using " + whichone + ", " + NMA_period + ", " + NMA_LB_min + ")"); IndicatorBuffers(3); SetIndexBuffer(0, NMC2); SetIndexLabel(0, "NMC2(" + NMC2_period + ", using " + whichone + ")"); SetIndexBuffer(1, NMC2_NMA); SetIndexLabel(1, "NMC2_NMA(" + NMA_period + ")"); SetIndexBuffer(2, NMC2_FastNMA); SetIndexLabel(2, "NMC2_FastNMA(" + NMA_period + ", " + NMA_LB_min + ")"); return(0); } int start() { int limit, i, ii, counted_bars = IndicatorCounted(); double nms, nmm, nmr, nms2, nmm2, nmr2, whichone, avg, sign, sum, abssum, ratio, nmc2num, maxnmc2; if(Bars <= NMC2_period) return(0); if(counted_bars < 0) counted_bars = 0; if(counted_bars > NMC2_period) limit = Bars - counted_bars; else limit = Bars - NMC2_period - 1; for(i = limit; i >= 0; i--) { nms2 = 0; nmm2 = 0; nmr2 = 0; for(ii = 1; ii <= NMC2_period; ii++) { double oLRSlope = 0, SumXY = 0, SumY = 0, SumX, SumXSqr, Divisor; if(ii > 1) { SumX = ii * (ii - 1) * 0.5; SumXSqr = ii * (ii - 1) * (2 * ii - 1) * 1/6; Divisor = (SumX * SumX) - ii * SumXSqr; for(int iii = 0; iii < ii; iii++) { SumXY += iii * MathLog(Close[i+iii]); SumY += MathLog(Close[i+iii]); } oLRSlope = (ii * SumXY - SumX * SumY) / Divisor; } nms2 += oLRSlope * MathSqrt(ii); nmm2 += (MathLog(Close[i]) - MathLog(Close[i+ii])) / MathSqrt(ii); nmr2 += (MathLog(Close[i+ii-1]) - MathLog(Close[i+ii])) * (MathSqrt(ii) - MathSqrt(ii-1)); } nms = nms2 * 100; nmm = (nmm2 / NMC2_period) * 1000; nmr = nmr2 * 1000; if(Use_NMR_instead_NMM) whichone = nmr; else whichone = nmm; avg = ((MathAbs(nms) * whichone) + (MathAbs(whichone) * nms)) / 2; if(avg > 0) sign = 1; else if(avg < 0) sign = -1; else sign = 0; NMC2[i] = sign * MathSqrt(MathAbs(avg)); } for(i = limit; i >= 0; i--) if(i < Bars - NMC2_period - 1 - NMA_period) { ratio = 0; sum = (NMC2[i] - NMC2[i+1]) + (NMC2[i+1] - NMC2[i+2]) * (MathSqrt(2)-1); for(ii = 2; ii < NMA_period; ii++) sum += (NMC2[i+ii] - NMC2[i+ii+1]) * (MathSqrt(ii+1) - MathSqrt(ii)); abssum = MathAbs(sum); sum = 0; for(ii = 0; ii < NMA_period; ii++) sum += MathAbs(NMC2[i+ii] - NMC2[i+ii+1]); if(sum != 0) ratio = abssum / sum; NMC2_NMA[i] = NMC2_NMA[i+1] + (NMC2[i] - NMC2_NMA[i+1]) * ratio; } for(i = limit; i >= 0; i--) if(i < Bars - NMC2_period - 1 - NMA_period) { maxnmc2 = 0; ratio = 0; int NMA_LB_max; for(ii = 1; ii <= NMA_period; ii++) { nmc2num = (NMC2[i] - NMC2[i+ii]) / MathSqrt(ii); if(MathAbs(nmc2num) > maxnmc2) { maxnmc2 = MathAbs(nmc2num); NMA_LB_max = ii; } } if(NMA_LB_max < NMA_LB_min) NMA_LB_max = NMA_LB_min; sum = (NMC2[i] - NMC2[i+1]) + (NMC2[i+1] - NMC2[i+2]) * (MathSqrt(2)-1); for(ii = 2; ii < NMA_LB_max; ii++) sum += (NMC2[i+ii] - NMC2[i+ii+1]) * (MathSqrt(ii+1) - MathSqrt(ii)); abssum = MathAbs(sum); sum = 0; for(ii = 0; ii < NMA_LB_max; ii++) sum += MathAbs(NMC2[i+ii] - NMC2[i+ii+1]); if(sum != 0) ratio = abssum / sum; NMC2_FastNMA[i] = NMC2_FastNMA[i+1] + (NMC2[i] - NMC2_FastNMA[i+1]) * ratio; } return(0); }
Â
The source code is also available for download here:
-
NMC2 for MT4
Â
NMC2.
Â
While NMC is calculated from NMM and NMR, NMC2 is calculated from NMS and either NMM or NMR, so you have two possible choices here.
To choose which one you want, set the option "Use_NMR_instead_NMM" either to "true" (for calculation using NMS and NMR) or to "false" (for calculation using NMS and NMM). Which variant was chosen can be then seen in the upper left corner of the indicator window.
Â
Â
Original NMC2 on TradeStation:
http://img337.imageshack.us/img337/87/nmc2tradestation.png
Â
NMC2 on Metatrader:
http://img220.imageshack.us/img220/1598/nmc2metatrader.png
Â
Original size screenshots:
http://img210.imageshack.us/img210/87/nmc2tradestation.png
http://img11.imageshack.us/img11/1598/nmc2metatrader.png
Â
#property indicator_separate_window #property indicator_buffers 2 #property indicator_color1 Magenta #property indicator_color2 Silver #property indicator_level1 0 #property indicator_levelcolor Gray #property indicator_levelstyle 2 extern int NMC2_period = 40; extern bool Use_NMR_instead_NMM = false; extern bool Show_SD = true; extern int SD_len = 30; extern double SD_up = 2; extern double SD_dn = 2; double NMC2[]; double SD[]; int init() { string whichone = "NMM"; if(Use_NMR_instead_NMM) whichone = "NMR"; string nmc2name = "NMC2(" + NMC2_period + ", using " + whichone + ")"; string sdname = "NMC2_SD(" + SD_len + ")"; IndicatorShortName(nmc2name); IndicatorBuffers(2); SetIndexBuffer(0, NMC2); SetIndexLabel(0, nmc2name); SetIndexBuffer(1, SD); SetIndexLabel(1, sdname); return(0); } int start() { int limit, i, ii, counted_bars = IndicatorCounted(); if(Bars <= NMC2_period) return(0); if(counted_bars < 0) counted_bars = 0; if(counted_bars > NMC2_period) limit = Bars - counted_bars; else limit = Bars - NMC2_period - 1; for(i = limit; i >= 0; i--) { double nms, nmm, nmr, nms2 = 0, nmm2 = 0, nmr2 = 0, whichone, avg, sign; for(ii = 1; ii <= NMC2_period; ii++) { double oLRSlope = 0, SumXY = 0, SumY = 0, SumX, SumXSqr, Divisor; if(ii > 1) { SumX = ii * (ii - 1) * 0.5; SumXSqr = ii * (ii - 1) * (2 * ii - 1) * 1/6; Divisor = (SumX * SumX) - ii * SumXSqr; for(int iii = 0; iii < ii; iii++) { SumXY += iii * MathLog(Close[i+iii]); SumY += MathLog(Close[i+iii]); } oLRSlope = (ii * SumXY - SumX * SumY) / Divisor; } nms2 += oLRSlope * MathSqrt(ii); nmm2 += (MathLog(Close[i]) - MathLog(Close[i+ii])) / MathSqrt(ii); nmr2 += (MathLog(Close[i+ii-1]) - MathLog(Close[i+ii])) * (MathSqrt(ii) - MathSqrt(ii-1)); } nms = nms2 * 100; nmm = (nmm2 / NMC2_period) * 1000; nmr = nmr2 * 1000; if(Use_NMR_instead_NMM) whichone = nmr; else whichone = nmm; avg = ((MathAbs(nms) * whichone) + (MathAbs(whichone) * nms)) / 2; if(avg > 0) sign = 1; else if(avg < 0) sign = -1; else sign = 0; NMC2[i] = sign * MathSqrt(MathAbs(avg)); } if(Show_SD) for(i = limit; i >= 0; i--) if(i < Bars - NMC2_period - 1 - SD_len) { if(NMC2[i] == 0) SD[i] = 0; else if(NMC2[i] > 0) SD[i] = iBandsOnArray(NMC2, 0, SD_len, SD_up, 0, MODE_UPPER, i); else if(NMC2[i] < 0) SD[i] = iBandsOnArray(NMC2, 0, SD_len, SD_dn, 0, MODE_LOWER, i); } return(0); }
Â
The source code is also available for download here:
- tgt123, indo37, damo1713005996 and 3 others
-
6
-
NMC_with_Ocn_MAs for MT4
Â
..and an older brother NMC_with_Ocn_MAs.
Â
Â
Original NMC_with_Ocn_MAs on TradeStation:
http://img43.imageshack.us/img43/6556/nmcwithocnmastradestati.png
Â
NMC_with_Ocn_MAs on Metatrader:
http://img220.imageshack.us/img220/7998/nmcwithocnmasmetatrader.png
Â
Original size screenshots:
http://img210.imageshack.us/img210/6556/nmcwithocnmastradestati.png
http://img822.imageshack.us/img822/7998/nmcwithocnmasmetatrader.png
Â
#property indicator_separate_window #property indicator_buffers 3 #property indicator_color1 Magenta #property indicator_color2 Yellow #property indicator_color3 Aqua #property indicator_level1 0 #property indicator_levelcolor Gray #property indicator_levelstyle 2 extern int NMC_period = 40; extern int NMA_period = 40; extern int NMA_LB_min = 8; double NMC[]; double NMC_NMA[]; double NMC_FastNMA[]; int init() { IndicatorShortName("NMC_with_Ocn_MAs(" + NMC_period + ", " + NMA_period + ", " + NMA_LB_min + ")"); IndicatorBuffers(3); SetIndexBuffer(0, NMC); SetIndexLabel(0, "NMC(" + NMC_period + ")"); SetIndexBuffer(1, NMC_NMA); SetIndexLabel(1, "NMC_NMA(" + NMA_period + ")"); SetIndexBuffer(2, NMC_FastNMA); SetIndexLabel(2, "NMC_FastNMA(" + NMA_period + ", " + NMA_LB_min + ")"); return(0); } int start() { int limit, i, ii, counted_bars = IndicatorCounted(); double nmm, nmr, nmm2, nmr2, avg, sign, sum, abssum, ratio, nmcnum, maxnmc; if(Bars <= NMC_period) return(0); if(counted_bars < 0) counted_bars = 0; if(counted_bars > NMC_period) limit = Bars - counted_bars; else limit = Bars - NMC_period - 1; for(i = limit; i >= 0; i--) { nmm2 = 0; nmr2 = 0; for(ii = 1; ii <= NMC_period; ii++) { nmm2 += (MathLog(Close[i]) - MathLog(Close[i+ii])) / MathSqrt(ii); nmr2 += (MathLog(Close[i+ii-1]) - MathLog(Close[i+ii])) * (MathSqrt(ii) - MathSqrt(ii-1)); } nmm = (nmm2 / NMC_period) * 1000; nmr = nmr2 * 1000; avg = ((MathAbs(nmm) * nmr) + (MathAbs(nmr) * nmm)) / 2; if(avg > 0) sign = 1; else if(avg < 0) sign = -1; else sign = 0; NMC[i] = sign * MathSqrt(MathAbs(avg)); } for(i = limit; i >= 0; i--) if(i < Bars - NMC_period - 1 - NMA_period) { ratio = 0; sum = (NMC[i] - NMC[i+1]) + (NMC[i+1] - NMC[i+2]) * (MathSqrt(2)-1); for(ii = 2; ii < NMA_period; ii++) sum += (NMC[i+ii] - NMC[i+ii+1]) * (MathSqrt(ii+1) - MathSqrt(ii)); abssum = MathAbs(sum); sum = 0; for(ii = 0; ii < NMA_period; ii++) sum += MathAbs(NMC[i+ii] - NMC[i+ii+1]); if(sum != 0) ratio = abssum / sum; NMC_NMA[i] = NMC_NMA[i+1] + (NMC[i] - NMC_NMA[i+1]) * ratio; } for(i = limit; i >= 0; i--) if(i < Bars - NMC_period - 1 - NMA_period) { maxnmc = 0; ratio = 0; int NMA_LB_max; for(ii = 1; ii <= NMA_period; ii++) { nmcnum = (NMC[i] - NMC[i+ii]) / MathSqrt(ii); if(MathAbs(nmcnum) > maxnmc) { maxnmc = MathAbs(nmcnum); NMA_LB_max = ii; } } if(NMA_LB_max < NMA_LB_min) NMA_LB_max = NMA_LB_min; sum = (NMC[i] - NMC[i+1]) + (NMC[i+1] - NMC[i+2]) * (MathSqrt(2)-1); for(ii = 2; ii < NMA_LB_max; ii++) sum += (NMC[i+ii] - NMC[i+ii+1]) * (MathSqrt(ii+1) - MathSqrt(ii)); abssum = MathAbs(sum); sum = 0; for(ii = 0; ii < NMA_LB_max; ii++) sum += MathAbs(NMC[i+ii] - NMC[i+ii+1]); if(sum != 0) ratio = abssum / sum; NMC_FastNMA[i] = NMC_FastNMA[i+1] + (NMC[i] - NMC_FastNMA[i+1]) * ratio; } return(0); }
Â
The source code is also available for download here:
- damo1713005996, kolikol, crodzilla and 4 others
-
7
-
NMC for MT4
Â
Long awaited NMC :)
Â
Â
Original NMC on TradeStation:
http://img849.imageshack.us/img849/961/nmctradestation.png
Â
NMC on Metatrader:
http://img341.imageshack.us/img341/1166/nmcmetatrader.png
Â
Original size screenshots:
http://img252.imageshack.us/img252/961/nmctradestation.png
http://img528.imageshack.us/img528/1166/nmcmetatrader.png
Â
#property indicator_separate_window #property indicator_buffers 2 #property indicator_color1 Magenta #property indicator_color2 Silver #property indicator_level1 0 #property indicator_levelcolor Gray #property indicator_levelstyle 2 extern int NMC_period = 40; extern bool Show_SD = true; extern int SD_len = 30; extern double SD_up = 2; extern double SD_dn = 2; double NMC[]; double SD[]; int init() { string nmcname = "NMC(" + NMC_period + ")"; string sdname = "NMC_SD(" + SD_len + ")"; IndicatorShortName(nmcname); IndicatorBuffers(2); SetIndexBuffer(0, NMC); SetIndexLabel(0, nmcname); SetIndexBuffer(1, SD); SetIndexLabel(1, sdname); return(0); } int start() { int limit, i, ii, counted_bars = IndicatorCounted(); if(Bars <= NMC_period) return(0); if(counted_bars < 0) counted_bars = 0; if(counted_bars > NMC_period) limit = Bars - counted_bars; else limit = Bars - NMC_period - 1; for(i = limit; i >= 0; i--) { double nmm, nmr, nmm2 = 0, nmr2 = 0, avg, sign; for(ii = 1; ii <= NMC_period; ii++) { nmm2 += (MathLog(Close[i]) - MathLog(Close[i+ii])) / MathSqrt(ii); nmr2 += (MathLog(Close[i+ii-1]) - MathLog(Close[i+ii])) * (MathSqrt(ii) - MathSqrt(ii-1)); } nmm = (nmm2 / NMC_period) * 1000; nmr = nmr2 * 1000; avg = ((MathAbs(nmm) * nmr) + (MathAbs(nmr) * nmm)) / 2; if(avg > 0) sign = 1; else if(avg < 0) sign = -1; else sign = 0; NMC[i] = sign * MathSqrt(MathAbs(avg)); } if(Show_SD) for(i = limit; i >= 0; i--) if(i < Bars - NMC_period - 1 - SD_len) { if(NMC[i] == 0) SD[i] = 0; else if(NMC[i] > 0) SD[i] = iBandsOnArray(NMC, 0, SD_len, SD_up, 0, MODE_UPPER, i); else if(NMC[i] < 0) SD[i] = iBandsOnArray(NMC, 0, SD_len, SD_dn, 0, MODE_LOWER, i); } return(0); }
Â
The source code is also available for download here:
-
NMM_ROC for MT4
Â
NMM_ROC - almost missed this one. Should be the last one from the NMM family.
Â
Â
Original NMM_ROC on TradeStation:
http://img72.imageshack.us/img72/5621/nmmroctradestation.png
Â
NMM_ROC on Metatrader:
http://img217.imageshack.us/img217/3605/nmmrocmetatrader.png
Â
Original size screenshots:
http://img18.imageshack.us/img18/5621/nmmroctradestation.png
http://img40.imageshack.us/img40/3605/nmmrocmetatrader.png
Â
#property indicator_separate_window #property indicator_buffers 2 #property indicator_color1 Magenta #property indicator_color2 Silver #property indicator_level1 0 #property indicator_levelcolor Gray #property indicator_levelstyle 2 extern int NMM_ROC_period = 40; extern int NMM_ROC_LB = 3; extern int NMM_ROC_AvgLen = 3; extern bool Show_SD = true; extern int SD_len = 30; extern double SD_up = 2; extern double SD_dn = 2; double NMM_ROC[]; double SD[]; double NMM[]; double NMM_ROCx[]; int init() { string nmmrocname = "NMM_ROC(" + NMM_ROC_period + ", " + NMM_ROC_LB + ", " + NMM_ROC_AvgLen + ")"; string sdname = "NMM_ROC_SD(" + SD_len + ")"; IndicatorShortName(nmmrocname); IndicatorBuffers(4); SetIndexBuffer(0, NMM_ROC); SetIndexLabel(0, nmmrocname); SetIndexBuffer(1, SD); SetIndexLabel(1, sdname); SetIndexBuffer(2, NMM); SetIndexBuffer(3, NMM_ROCx); return(0); } int start() { int limit, i, ii, counted_bars = IndicatorCounted(); if(Bars <= NMM_ROC_period + NMM_ROC_LB + NMM_ROC_AvgLen) return(0); if(counted_bars < 0) counted_bars = 0; if(counted_bars > NMM_ROC_period) limit = Bars - counted_bars; else limit = Bars - NMM_ROC_period - 1; for(i = limit; i >= 0; i--) { double nmm2 = 0; for(ii = 1; ii <= NMM_ROC_period; ii++) nmm2 += (MathLog(Close[i]) - MathLog(Close[i+ii])) / MathSqrt(ii); NMM[i] = (nmm2 / NMM_ROC_period) * 1000; } for(i = limit; i >= 0; i--) if(i < Bars - NMM_ROC_period - 1 - NMM_ROC_LB) NMM_ROCx[i] = NMM[i] - NMM[i+NMM_ROC_LB]; for(i = limit; i >= 0; i--) if(i < Bars - NMM_ROC_period - 1 - NMM_ROC_LB - NMM_ROC_AvgLen) { double WtdSum = 0; for(ii = 0; ii < NMM_ROC_AvgLen; ii++) WtdSum += (NMM_ROC_AvgLen - ii) * NMM_ROCx[i+ii]; NMM_ROC[i] = WtdSum / ((NMM_ROC_AvgLen + 1) * NMM_ROC_AvgLen * 0.5); } if(Show_SD) for(i = limit; i >= 0; i--) if(i < Bars - NMM_ROC_period - 1 - NMM_ROC_LB - NMM_ROC_AvgLen - SD_len) { if(NMM_ROC[i] == 0) SD[i] = 0; else if(NMM_ROC[i] > 0) SD[i] = iBandsOnArray(NMM_ROC, 0, SD_len, SD_up, 0, MODE_UPPER, i); else if(NMM_ROC[i] < 0) SD[i] = iBandsOnArray(NMM_ROC, 0, SD_len, SD_dn, 0, MODE_LOWER, i); } return(0); }
Â
The source code is also available for download here:
- damo1713005996, C0UNDE, indo37 and 5 others
-
8
-
NMM_MACD_with_Ocn_MAs for MT4
Â
NMM_MACD_with_Ocn_MAs. Again bug in FastNMA TS source code.
Â
Now the 3 lines in the chart are calculated like this:
magenta: Price -> NMM -> NMA -> (NMM-NMA) yellow: Price -> NMM -> NMA -> (NMM-NMA) -> NMA blue: Price -> NMM -> NMA -> (NMM-NMA) -> FastNMA
Â
Â
Original NMM_MACD_with_Ocn_MAs on TradeStation:
http://img713.imageshack.us/img713/6022/nmmmacdwithocnmastrades.png
Â
NMM_MACD_with_Ocn_MAs on Metatrader:
http://img26.imageshack.us/img26/7956/nmmmacdwithocnmasmetatr.png
Â
Original size screenshots:
http://img194.imageshack.us/img194/6022/nmmmacdwithocnmastrades.png
http://img140.imageshack.us/img140/7956/nmmmacdwithocnmasmetatr.png
Â
#property indicator_separate_window #property indicator_buffers 3 #property indicator_color1 Magenta #property indicator_color2 Yellow #property indicator_color3 Aqua #property indicator_level1 0 #property indicator_levelcolor Gray #property indicator_levelstyle 2 extern int NMM_MACD_period = 40; extern int NMA_period = 40; extern int NMA_LB_min = 8; double NMM_MACD[]; double NMM_MACD_NMA[]; double NMM_MACD_FastNMA[]; double NMM[]; double NMM_MA[]; int init() { IndicatorShortName("NMM_MACD_with_Ocn_MAs(" + NMM_MACD_period + ", " + NMA_period + ", " + NMA_LB_min + ")"); IndicatorBuffers(5); SetIndexBuffer(0, NMM_MACD); SetIndexLabel(0, "NMM_MACD(" + NMM_MACD_period + ")"); SetIndexBuffer(1, NMM_MACD_NMA); SetIndexLabel(1, "NMM_MACD_NMA(" + NMA_period + ")"); SetIndexBuffer(2, NMM_MACD_FastNMA); SetIndexLabel(2, "NMM_MACD_FastNMA(" + NMA_period + ", " + NMA_LB_min + ")"); SetIndexBuffer(3, NMM); SetIndexBuffer(4, NMM_MA); return(0); } int start() { int limit, i, ii, counted_bars = IndicatorCounted(); double nmm2, sum, abssum, ratio, nmmnum, maxnmm; if(Bars <= 2*NMM_MACD_period) return(0); if(counted_bars < 0) counted_bars = 0; if(counted_bars > NMM_MACD_period) limit = Bars - counted_bars; else limit = Bars - NMM_MACD_period - 1; for(i = limit; i >= 0; i--) { nmm2 = 0; for(ii = 1; ii <= NMM_MACD_period; ii++) nmm2 += (MathLog(Close[i]) - MathLog(Close[i+ii])) / MathSqrt(ii); NMM[i] = (nmm2 / NMM_MACD_period) * 1000; } for(i = limit; i >= 0; i--) if(i < Bars - 2*NMM_MACD_period - 1) { ratio = 0; sum = (NMM[i] - NMM[i+1]) + (NMM[i+1] - NMM[i+2]) * (MathSqrt(2)-1); for(ii = 2; ii < NMM_MACD_period; ii++) sum += (NMM[i+ii] - NMM[i+ii+1]) * (MathSqrt(ii+1) - MathSqrt(ii)); abssum = MathAbs(sum); sum = 0; for(ii = 0; ii < NMM_MACD_period; ii++) sum += MathAbs(NMM[i+ii] - NMM[i+ii+1]); if(sum != 0) ratio = abssum / sum; NMM_MA[i] = NMM_MA[i+1] + (NMM[i] - NMM_MA[i+1]) * ratio; NMM_MACD[i] = NMM[i] - NMM_MA[i]; } for(i = limit; i >= 0; i--) if(i < Bars - 2*NMM_MACD_period - 1 - NMA_period) { ratio = 0; sum = (NMM_MACD[i] - NMM_MACD[i+1]) + (NMM_MACD[i+1] - NMM_MACD[i+2]) * (MathSqrt(2)-1); for(ii = 2; ii < NMA_period; ii++) sum += (NMM_MACD[i+ii] - NMM_MACD[i+ii+1]) * (MathSqrt(ii+1) - MathSqrt(ii)); abssum = MathAbs(sum); sum = 0; for(ii = 0; ii < NMA_period; ii++) sum += MathAbs(NMM_MACD[i+ii] - NMM_MACD[i+ii+1]); if(sum != 0) ratio = abssum / sum; NMM_MACD_NMA[i] = NMM_MACD_NMA[i+1] + (NMM_MACD[i] - NMM_MACD_NMA[i+1]) * ratio; } for(i = limit; i >= 0; i--) if(i < Bars - 2*NMM_MACD_period - 1 - NMA_period) { maxnmm = 0; ratio = 0; int NMA_LB_max; for(ii = 1; ii <= NMA_period; ii++) { nmmnum = (NMM_MACD[i] - NMM_MACD[i+ii]) / MathSqrt(ii); if(MathAbs(nmmnum) > maxnmm) { maxnmm = MathAbs(nmmnum); NMA_LB_max = ii; } } if(NMA_LB_max < NMA_LB_min) NMA_LB_max = NMA_LB_min; sum = (NMM_MACD[i] - NMM_MACD[i+1]) + (NMM_MACD[i+1] - NMM_MACD[i+2]) * (MathSqrt(2)-1); for(ii = 2; ii < NMA_LB_max; ii++) sum += (NMM_MACD[i+ii] - NMM_MACD[i+ii+1]) * (MathSqrt(ii+1) - MathSqrt(ii)); abssum = MathAbs(sum); sum = 0; for(ii = 0; ii < NMA_LB_max; ii++) sum += MathAbs(NMM_MACD[i+ii] - NMM_MACD[i+ii+1]); if(sum != 0) ratio = abssum / sum; NMM_MACD_FastNMA[i] = NMM_MACD_FastNMA[i+1] + (NMM_MACD[i] - NMM_MACD_FastNMA[i+1]) * ratio; } return(0); }
Â
The source code is also available for download here:
Â
Â
Next one will be NMC.
-
NMM_MACD for MT4
Â
This one is NMM_MACD. First it calculates NMM, then it calculates NMA of it (like in NMM_with_Ocn_MAs), but what is actually drawn on the chart is the difference between those two, i.e. NMM-NMA.
Â
Â
Original NMM_MACD on TradeStation:
http://img585.imageshack.us/img585/1374/nmmmacdtradestation.png
Â
NMM_MACD on Metatrader:
http://img844.imageshack.us/img844/7105/nmmmacdmetatrader.png
Â
Original size screenshots:
http://img834.imageshack.us/img834/1374/nmmmacdtradestation.png
http://img191.imageshack.us/img191/7105/nmmmacdmetatrader.png
Â
#property indicator_separate_window #property indicator_buffers 2 #property indicator_color1 Magenta #property indicator_color2 Silver #property indicator_level1 0 #property indicator_levelcolor Gray #property indicator_levelstyle 2 extern int NMM_MACD_period = 40; extern bool Show_SD = true; extern int SD_len = 30; extern double SD_up = 2; extern double SD_dn = 2; double NMM_MACD[]; double SD[]; double NMM[]; double NMM_MA[]; int init() { string nmmmacdname = "NMM_MACD(" + NMM_MACD_period + ")"; string sdname = "NMM_MACD_SD(" + SD_len + ")"; IndicatorShortName(nmmmacdname); IndicatorBuffers(4); SetIndexBuffer(0, NMM_MACD); SetIndexLabel(0, nmmmacdname); SetIndexBuffer(1, SD); SetIndexLabel(1, sdname); SetIndexBuffer(2, NMM); SetIndexBuffer(3, NMM_MA); return(0); } int start() { int limit, i, ii, counted_bars = IndicatorCounted(); double nmm2, sum, abssum, ratio; if(Bars <= 2*NMM_MACD_period) return(0); if(counted_bars < 0) counted_bars = 0; if(counted_bars > NMM_MACD_period) limit = Bars - counted_bars; else limit = Bars - NMM_MACD_period - 1; for(i = limit; i >= 0; i--) { nmm2 = 0; for(ii = 1; ii <= NMM_MACD_period; ii++) nmm2 += (MathLog(Close[i]) - MathLog(Close[i+ii])) / MathSqrt(ii); NMM[i] = (nmm2 / NMM_MACD_period) * 1000; } for(i = limit; i >= 0; i--) if(i < Bars - 2*NMM_MACD_period - 1) { ratio = 0; sum = (NMM[i] - NMM[i+1]) + (NMM[i+1] - NMM[i+2]) * (MathSqrt(2)-1); for(ii = 2; ii < NMM_MACD_period; ii++) sum += (NMM[i+ii] - NMM[i+ii+1]) * (MathSqrt(ii+1) - MathSqrt(ii)); abssum = MathAbs(sum); sum = 0; for(ii = 0; ii < NMM_MACD_period; ii++) sum += MathAbs(NMM[i+ii] - NMM[i+ii+1]); if(sum != 0) ratio = abssum / sum; NMM_MA[i] = NMM_MA[i+1] + (NMM[i] - NMM_MA[i+1]) * ratio; NMM_MACD[i] = NMM[i] - NMM_MA[i]; } if(Show_SD) for(i = limit; i >= 0; i--) if(i < Bars - 2*NMM_MACD_period - 1 - SD_len) { if(NMM_MACD[i] == 0) SD[i] = 0; else if(NMM_MACD[i] > 0) SD[i] = iBandsOnArray(NMM_MACD, 0, SD_len, SD_up, 0, MODE_UPPER, i); else if(NMM_MACD[i] < 0) SD[i] = iBandsOnArray(NMM_MACD, 0, SD_len, SD_dn, 0, MODE_LOWER, i); } return(0); }
Â
The source code is also available for download here:
- ahuramazdi, kolikol, crodzilla and 6 others
-
9
-
NMS_with_Ocn_MAs for MT4
Â
NMS_with_Ocn_MAs.
Â
There is again a bug in the TradeStation source code regarding the FastNMA's lookback calculation. Actually it's in all source codes where NMA & FastNMA are applied onto another Ocean indi.
Â
Wherever you see a line like this:
Value3 = _Ocn.FastMA.Fn (FALSE, Value1, CLOSE, SC, 1, 1, LB.Min) ;
Â
the third parameter of _Ocn.FastMA.Fn must be identical to the second parameter, so it must be like this:
Value3 = _Ocn.FastMA.Fn (FALSE, Value1, Value1, SC, 1, 1, LB.Min) ;
Â
Â
Original NMS_with_Ocn_MAs on TradeStation:
http://img208.imageshack.us/img208/7532/nmswithocnmastradestati.png
Â
NMS_with_Ocn_MAs on Metatrader:
http://img140.imageshack.us/img140/6875/nmswithocnmasmetatrader.png
Â
Original size screenshots:
http://img38.imageshack.us/img38/7532/nmswithocnmastradestati.png
http://img525.imageshack.us/img525/6875/nmswithocnmasmetatrader.png
Â
#property indicator_separate_window #property indicator_buffers 3 #property indicator_color1 Magenta #property indicator_color2 Yellow #property indicator_color3 Aqua #property indicator_level1 0 #property indicator_levelcolor Gray #property indicator_levelstyle 2 extern int NMS_period = 40; extern int NMA_period = 40; extern int NMA_LB_min = 8; double NMS[]; double NMS_NMA[]; double NMS_FastNMA[]; int init() { IndicatorShortName("NMS_with_Ocn_MAs(" + NMS_period + ", " + NMA_period + ", " + NMA_LB_min + ")"); IndicatorBuffers(3); SetIndexBuffer(0, NMS); SetIndexLabel(0, "NMS(" + NMS_period + ")"); SetIndexBuffer(1, NMS_NMA); SetIndexLabel(1, "NMS_NMA(" + NMA_period + ")"); SetIndexBuffer(2, NMS_FastNMA); SetIndexLabel(2, "NMS_FastNMA(" + NMA_period + ", " + NMA_LB_min + ")"); return(0); } int start() { int limit, i, ii, counted_bars = IndicatorCounted(); double nms2, sum, abssum, ratio, nmsnum, maxnms; if(Bars <= NMS_period) return(0); if(counted_bars < 0) counted_bars = 0; if(counted_bars > NMS_period) limit = Bars - counted_bars; else limit = Bars - NMS_period - 1; for(i = limit; i >= 0; i--) { nms2 = 0; for(ii = 1; ii <= NMS_period; ii++) { double oLRSlope = 0, SumXY = 0, SumY = 0, SumX, SumXSqr, Divisor; if(ii > 1) { SumX = ii * (ii - 1) * 0.5; SumXSqr = ii * (ii - 1) * (2 * ii - 1) * 1/6; Divisor = (SumX * SumX) - ii * SumXSqr; for(int iii = 0; iii < ii; iii++) { SumXY += iii * MathLog(Close[i+iii]); SumY += MathLog(Close[i+iii]); } oLRSlope = (ii * SumXY - SumX * SumY) / Divisor; } nms2 += oLRSlope * MathSqrt(ii); } NMS[i] = nms2 * 100; } for(i = limit; i >= 0; i--) if(i < Bars - NMS_period - 1 - NMA_period) { ratio = 0; sum = (NMS[i] - NMS[i+1]) + (NMS[i+1] - NMS[i+2]) * (MathSqrt(2)-1); for(ii = 2; ii < NMA_period; ii++) sum += (NMS[i+ii] - NMS[i+ii+1]) * (MathSqrt(ii+1) - MathSqrt(ii)); abssum = MathAbs(sum); sum = 0; for(ii = 0; ii < NMA_period; ii++) sum += MathAbs(NMS[i+ii] - NMS[i+ii+1]); if(sum != 0) ratio = abssum / sum; NMS_NMA[i] = NMS_NMA[i+1] + (NMS[i] - NMS_NMA[i+1]) * ratio; } for(i = limit; i >= 0; i--) if(i < Bars - NMS_period - 1 - NMA_period) { maxnms = 0; ratio = 0; int NMA_LB_max; for(ii = 1; ii <= NMA_period; ii++) { nmsnum = (NMS[i] - NMS[i+ii]) / MathSqrt(ii); if(MathAbs(nmsnum) > maxnms) { maxnms = MathAbs(nmsnum); NMA_LB_max = ii; } } if(NMA_LB_max < NMA_LB_min) NMA_LB_max = NMA_LB_min; sum = (NMS[i] - NMS[i+1]) + (NMS[i+1] - NMS[i+2]) * (MathSqrt(2)-1); for(ii = 2; ii < NMA_LB_max; ii++) sum += (NMS[i+ii] - NMS[i+ii+1]) * (MathSqrt(ii+1) - MathSqrt(ii)); abssum = MathAbs(sum); sum = 0; for(ii = 0; ii < NMA_LB_max; ii++) sum += MathAbs(NMS[i+ii] - NMS[i+ii+1]); if(sum != 0) ratio = abssum / sum; NMS_FastNMA[i] = NMS_FastNMA[i+1] + (NMS[i] - NMS_FastNMA[i+1]) * ratio; } return(0); }
Â
The source code is also available for download here:
-
NMS for MT4
Â
NMS.
Â
It's slowly getting more interesting.
Â
Â
Original NMS on TradeStation:
http://img29.imageshack.us/img29/3972/nmstradestation.png
Â
NMS on Metatrader:
http://img525.imageshack.us/img525/6312/nmsmetatrader.png
Â
Original size screenshots:
http://img526.imageshack.us/img526/3972/nmstradestation.png
http://img585.imageshack.us/img585/6312/nmsmetatrader.png
Â
#property indicator_separate_window #property indicator_buffers 2 #property indicator_color1 Magenta #property indicator_color2 Silver #property indicator_level1 0 #property indicator_levelcolor Gray #property indicator_levelstyle 2 extern int NMS_period = 40; extern bool Show_SD = true; extern int SD_len = 30; extern double SD_up = 2; extern double SD_dn = 2; double NMS[]; double SD[]; int init() { string nmsname = "NMS(" + NMS_period + ")"; string sdname = "NMS_SD(" + SD_len + ")"; IndicatorShortName(nmsname); IndicatorBuffers(2); SetIndexBuffer(0, NMS); SetIndexLabel(0, nmsname); SetIndexBuffer(1, SD); SetIndexLabel(1, sdname); return(0); } int start() { int limit, i, ii, counted_bars = IndicatorCounted(); if(Bars <= NMS_period) return(0); if(counted_bars < 0) counted_bars = 0; if(counted_bars > NMS_period) limit = Bars - counted_bars; else limit = Bars - NMS_period - 1; for(i = limit; i >= 0; i--) { double nms2 = 0; for(ii = 1; ii <= NMS_period; ii++) { double oLRSlope = 0, SumXY = 0, SumY = 0, SumX, SumXSqr, Divisor; if(ii > 1) { SumX = ii * (ii - 1) * 0.5; SumXSqr = ii * (ii - 1) * (2 * ii - 1) * 1/6; Divisor = (SumX * SumX) - ii * SumXSqr; for(int iii = 0; iii < ii; iii++) { SumXY += iii * MathLog(Close[i+iii]); SumY += MathLog(Close[i+iii]); } oLRSlope = (ii * SumXY - SumX * SumY) / Divisor; } nms2 += oLRSlope * MathSqrt(ii); } NMS[i] = nms2 * 100; } if(Show_SD) for(i = limit; i >= 0; i--) if(i < Bars - NMS_period - 1 - SD_len) { if(NMS[i] == 0) SD[i] = 0; else if(NMS[i] > 0) SD[i] = iBandsOnArray(NMS, 0, SD_len, SD_up, 0, MODE_UPPER, i); else if(NMS[i] < 0) SD[i] = iBandsOnArray(NMS, 0, SD_len, SD_dn, 0, MODE_LOWER, i); } return(0); }
Â
The source code is also available for download here:
Â
Â
fawaz79: I will get to NMC too, just patience.
- tgt123, ahuramazdi, kolikol and 7 others
-
10
-
The file "Ocean Indicators Package (EL Code).els" is in the parent folder of where the source code files are. There are also 4 manual PDF files in that folder, you can't miss it. Just double-click the .els file and import all indicators and functions into TS.
-
Grain Trader: I posted all the links in this post:
-
Well, the the package I posted here does include "Ocean Plus Indicator Package" which contains "BTX_1Line w SD", "BTX_2Line w SD" and "STX" studies and supporting functions. Whether these BTX and STX are what you are referring to or they are some "lesser" versions, I don't know. Anyway, the logic in these is moved to the external DLL which is PC-Guard 5.0 protected. That means that the actual algorithm may be hard to get, but it still could be possible to get those on the Metatrader platform.
-
monstar: that's strange. Only reason why it wouldn't show anything would be if you attached it to a chart which got less than 40 bars of history. But that's hardly the case (if it was the case you can simply hold "Home" key until it loads more bars and then attach the indi again to the chart).
Â
You can also check the "Experts" tab of the "Terminal" window, there may be some error message shown:
Â
http://articles.mql4.com/c/articles/2009/02/expert.png
Â
Â
Anyway, test the indis, guys, as I may make a mistake or overlook something. If there is anything wrong or if it doesn't show exactly the same as the TradeStation version (except the discrepancies due to the different price feed), just shout or something :)
Â
Right now I am at 15:38 in the DVD3 video, Pat is explaining the NMS so that will be the next one I guess. It got me a bit angry, though, because NMS uses a linear regression slope function and in TradeStation that's a built-in function, in Metatrader I will have to reprogram it from the scratch.
Â
This is my first experience with TradeStation, I never even tried it before, and I must say it's incomparable to Metatrader. Metatrader to TradeStation is like Windows to Unix. It's got modern look and feel, it's intuitive and what not, but inside it's just dull. There are only as many functions and features as necessary whereas TradeStation is loaded. If it wasn't for the Metatrader Server's virtual dealer plug-in it would probably never got spreaded out among all those bucketshops, a.k.a. retail forex brokers/dealers/scammers/whatever-you-call-them, and consequently among the traders. Oh, well..
-
Reupload of the manual posted here and of the content of these torrents:
http://thepiratebay.se/torrent/6943545/MetaTrader_-_Virtual_Dealer_Plug_In__Video_by_ECNJesus
http://thepiratebay.se/torrent/7066441/Virtual_Dealer_Plugin_Manual
Â
Â
http://www.ulozto.net/xxXrKXE/metatrader-virtual-dealer-plugin-pdf
http://www.ulozto.net/live/xsnzpLd/ecnjesus-mp4
http://www.ulozto.net/xrW19Gg/metatraderadmin-chm
-
NMM_with_Ocn_MAs for MT4
Â
NMM_with_Ocn_MAs.
Â
This one is the NMM indi as before, but instead of showing SD bands there are NMA and FastNMA applied onto NMM.
Â
The reason why FastNMA of NMM is different in my implementation is because there is a mistake in the original TradeStation version. When Pat was modifying the FastNMA code to take NMM as an input (instead of the price) he forgot to change the input for lookback calculation so he is still calculating the lookback from the price.
Â
For those interested in TradeStation version, the following change needs to be done in the $NMM_with Ocn MAs study:
Â
(wrong)
Value1 = _Ocn.NMM.Fn (Price, SC, 1, 1) ; Value2 = _Ocn.MA.Fn (FALSE, Value1, 1) ; Value3 = _Ocn.FastMA.Fn (FALSE, Value1, [b][u]CLOSE[/u][/b], SC, 1, 1, LB.Min) ;
(correct)
Value1 = _Ocn.NMM.Fn (Price, SC, 1, 1) ; Value2 = _Ocn.MA.Fn (FALSE, Value1, 1) ; Value3 = _Ocn.FastMA.Fn (FALSE, Value1, [b][u]Value1[/u][/b], SC, 1, 1, LB.Min) ;
Â
Â
Original NMM_with_Ocn_MAs on TradeStation:
http://img827.imageshack.us/img827/3440/nmmwithocnmastradestati.png
Â
NMM_with_Ocn_MAs on Metatrader:
http://img6.imageshack.us/img6/3783/nmmwithocnmasmetatrader.png
Â
Original size screenshots:
http://img542.imageshack.us/img542/3440/nmmwithocnmastradestati.png
http://img820.imageshack.us/img820/3783/nmmwithocnmasmetatrader.png
Â
#property indicator_separate_window #property indicator_buffers 3 #property indicator_color1 Magenta #property indicator_color2 Yellow #property indicator_color3 Aqua #property indicator_level1 0 #property indicator_levelcolor Gray #property indicator_levelstyle 2 extern int NMM_period = 40; extern int NMA_period = 40; extern int NMA_LB_min = 8; double NMM[]; double NMM_NMA[]; double NMM_FastNMA[]; int init() { IndicatorShortName("NMM_with_Ocn_MAs(" + NMM_period + ", " + NMA_period + ", " + NMA_LB_min + ")"); IndicatorBuffers(3); SetIndexBuffer(0, NMM); SetIndexLabel(0, "NMM(" + NMM_period + ")"); SetIndexBuffer(1, NMM_NMA); SetIndexLabel(1, "NMM_NMA(" + NMA_period + ")"); SetIndexBuffer(2, NMM_FastNMA); SetIndexLabel(2, "NMM_FastNMA(" + NMA_period + ", " + NMA_LB_min + ")"); return(0); } int start() { int limit, i, ii, counted_bars = IndicatorCounted(); double nmm2, sum, abssum, ratio, nmmnum, maxnmm; if(Bars <= NMM_period) return(0); if(counted_bars < 0) counted_bars = 0; if(counted_bars > NMM_period) limit = Bars - counted_bars; else limit = Bars - NMM_period - 1; for(i = limit; i >= 0; i--) { nmm2 = 0; for(ii = 1; ii <= NMM_period; ii++) nmm2 += (MathLog(Close[i]) - MathLog(Close[i+ii])) / MathSqrt(ii); NMM[i] = (nmm2 / NMM_period) * 1000; } for(i = limit; i >= 0; i--) if(i < Bars - NMM_period - 1 - NMA_period) { ratio = 0; sum = (NMM[i] - NMM[i+1]) + (NMM[i+1] - NMM[i+2]) * (MathSqrt(2)-1); for(ii = 2; ii < NMA_period; ii++) sum += (NMM[i+ii] - NMM[i+ii+1]) * (MathSqrt(ii+1) - MathSqrt(ii)); abssum = MathAbs(sum); sum = 0; for(ii = 0; ii < NMA_period; ii++) sum += MathAbs(NMM[i+ii] - NMM[i+ii+1]); if(sum != 0) ratio = abssum / sum; NMM_NMA[i] = NMM_NMA[i+1] + (NMM[i] - NMM_NMA[i+1]) * ratio; } for(i = limit; i >= 0; i--) if(i < Bars - NMM_period - 1 - NMA_period) { maxnmm = 0; ratio = 0; int NMA_LB_max; for(ii = 1; ii <= NMA_period; ii++) { nmmnum = (NMM[i] - NMM[i+ii]) / MathSqrt(ii); if(MathAbs(nmmnum) > maxnmm) { maxnmm = MathAbs(nmmnum); NMA_LB_max = ii; } } if(NMA_LB_max < NMA_LB_min) NMA_LB_max = NMA_LB_min; sum = (NMM[i] - NMM[i+1]) + (NMM[i+1] - NMM[i+2]) * (MathSqrt(2)-1); for(ii = 2; ii < NMA_LB_max; ii++) sum += (NMM[i+ii] - NMM[i+ii+1]) * (MathSqrt(ii+1) - MathSqrt(ii)); abssum = MathAbs(sum); sum = 0; for(ii = 0; ii < NMA_LB_max; ii++) sum += MathAbs(NMM[i+ii] - NMM[i+ii+1]); if(sum != 0) ratio = abssum / sum; NMM_FastNMA[i] = NMM_FastNMA[i+1] + (NMM[i] - NMM_FastNMA[i+1]) * ratio; } return(0); }
Â
The source code is also available for download here:
Â
Â
tgt123: you are welcome.
- C0UNDE, monstar, Grain Trader and 3 others
-
6
-
NMA_Price_Osc for MT4
Â
NMA_Price_Osc.
Â
This indi shows the distance between the price and NMA in the form of an oscilator. The TradeStation's original version can do that only for the regular NMA. My Metatrader version can do that also for the Fast NMA.
Â
Â
Original NMA_Price_Osc on TradeStation:
http://img252.imageshack.us/img252/4949/nmapriceosctradestation.png
Â
NMA_Price_Osc on Metatrader:
http://img851.imageshack.us/img851/8864/nmapriceoscmetatrader.png
Â
Original size screenshots:
http://img39.imageshack.us/img39/4949/nmapriceosctradestation.png
http://img560.imageshack.us/img560/8864/nmapriceoscmetatrader.png
Â
#property indicator_separate_window #property indicator_buffers 2 #property indicator_color1 Magenta #property indicator_color2 Silver #property indicator_level1 0 #property indicator_levelcolor Gray #property indicator_levelstyle 2 extern int NMA_period = 40; extern bool FastNMA = true; extern int NMA_LB_min = 8; extern bool Show_SD = true; extern int SD_len = 30; extern double SD_up = 2; extern double SD_dn = 2; double Price_Osc[]; double SD[]; double NMA[]; int init() { string nmaname = "NMA_Price_Osc(" + NMA_period + ")"; if(FastNMA) nmaname = "Fast_NMA_Price_Osc(" + NMA_period + ", " + NMA_LB_min + ")"; string sdname = "NMA_Price_Osc_SD(" + SD_len + ")"; IndicatorShortName(nmaname); IndicatorBuffers(3); SetIndexBuffer(0, Price_Osc); SetIndexLabel(0, nmaname); SetIndexBuffer(1, SD); SetIndexLabel(1, sdname); SetIndexBuffer(2, NMA); return(0); } int start() { int limit, i, ii, counted_bars = IndicatorCounted(); if(Bars <= NMA_period) return(0); if(counted_bars < 0) counted_bars = 0; if(counted_bars > NMA_period) limit = Bars - counted_bars; else limit = Bars - NMA_period - 1; for(i = limit; i >= 0; i--) { double nmmnum, maxnmm = 0, sum, abssum, ratio = 0; int NMA_LB_max; if(FastNMA) { for(ii = 1; ii <= NMA_period; ii++) { nmmnum = (MathLog(Close[i]) - MathLog(Close[i+ii])) / MathSqrt(ii); if(MathAbs(nmmnum) > maxnmm) { maxnmm = MathAbs(nmmnum); NMA_LB_max = ii; } } if(NMA_LB_max < NMA_LB_min) NMA_LB_max = NMA_LB_min; } else NMA_LB_max = NMA_period; sum = (MathLog(Close[i]) - MathLog(Close[i+1])) + (MathLog(Close[i+1]) - MathLog(Close[i+2])) * (MathSqrt(2)-1); for(ii = 2; ii < NMA_LB_max; ii++) sum += (MathLog(Close[i+ii]) - MathLog(Close[i+ii+1])) * (MathSqrt(ii+1) - MathSqrt(ii)); abssum = MathAbs(sum); sum = 0; for(ii = 0; ii < NMA_LB_max; ii++) sum += MathAbs(MathLog(Close[i+ii]) - MathLog(Close[i+ii+1])); if(sum != 0) ratio = abssum / sum; NMA[i] = NMA[i+1] + (Close[i] - NMA[i+1]) * ratio; Price_Osc[i] = Close[i] - NMA[i]; } if(Show_SD) for(i = limit; i >= 0; i--) if(i < Bars - NMA_period - 1 - SD_len) { if(Price_Osc[i] == 0) SD[i] = 0; else if(Price_Osc[i] > 0) SD[i] = iBandsOnArray(Price_Osc, 0, SD_len, SD_up, 0, MODE_UPPER, i); else if(Price_Osc[i] < 0) SD[i] = iBandsOnArray(Price_Osc, 0, SD_len, SD_dn, 0, MODE_LOWER, i); } return(0); }
Â
The source code is also available for download here:
-
NMA_SD_Band_Osc for MT4
Â
NMA_SD_Band_Osc.
Â
Â
Original NMA_SD_Band_Osc on TradeStation:
http://img13.imageshack.us/img13/7295/nmasdbandosctradestatio.png
Â
NMA_SD_Band_Osc on Metatrader:
http://img836.imageshack.us/img836/6674/nmasdbandoscmetatrader.png
Â
Original size screenshots:
http://img526.imageshack.us/img526/7295/nmasdbandosctradestatio.png
http://img401.imageshack.us/img401/6674/nmasdbandoscmetatrader.png
Â
#property indicator_separate_window #property indicator_buffers 1 #property indicator_color1 Red #property indicator_level1 0 #property indicator_level2 50 #property indicator_level3 100 #property indicator_levelcolor Gray #property indicator_levelstyle 2 extern int NMA_period = 40; extern bool FastNMA = true; extern int NMA_LB_min = 8; extern int SD_len = 20; extern double SD_up = 1.5; extern double SD_dn = 1.5; double SD_Band_Osc[]; double NMA[]; double SDup[]; double SDdn[]; int init() { string nmaname = "NMA_SD_Band_Osc(" + NMA_period + ")"; if(FastNMA) nmaname = "Fast_NMA_SD_Band_Osc(" + NMA_period + ", " + NMA_LB_min + ")"; IndicatorShortName(nmaname); IndicatorBuffers(4); SetIndexBuffer(0, SD_Band_Osc); SetIndexLabel(0, nmaname); SetIndexBuffer(1, NMA); SetIndexBuffer(2, SDup); SetIndexBuffer(3, SDdn); return(0); } int start() { int limit, i, ii, counted_bars = IndicatorCounted(); if(Bars <= MathMax(NMA_period, SD_len)) return(0); if(counted_bars < 0) counted_bars = 0; if(counted_bars > MathMax(NMA_period, SD_len)) limit = Bars - counted_bars; else limit = Bars - MathMax(NMA_period, SD_len) - 1; for(i = limit; i >= 0; i--) { double nmmnum, maxnmm = 0, sum, abssum, ratio = 0; int NMA_LB_max; if(FastNMA) { for(ii = 1; ii <= NMA_period; ii++) { nmmnum = (MathLog(Close[i]) - MathLog(Close[i+ii])) / MathSqrt(ii); if(MathAbs(nmmnum) > maxnmm) { maxnmm = MathAbs(nmmnum); NMA_LB_max = ii; } } if(NMA_LB_max < NMA_LB_min) NMA_LB_max = NMA_LB_min; } else NMA_LB_max = NMA_period; sum = (MathLog(Close[i]) - MathLog(Close[i+1])) + (MathLog(Close[i+1]) - MathLog(Close[i+2])) * (MathSqrt(2)-1); for(ii = 2; ii < NMA_LB_max; ii++) sum += (MathLog(Close[i+ii]) - MathLog(Close[i+ii+1])) * (MathSqrt(ii+1) - MathSqrt(ii)); abssum = MathAbs(sum); sum = 0; for(ii = 0; ii < NMA_LB_max; ii++) sum += MathAbs(MathLog(Close[i+ii]) - MathLog(Close[i+ii+1])); if(sum != 0) ratio = abssum / sum; NMA[i] = NMA[i+1] + (Close[i] - NMA[i+1]) * ratio; SDup[i] = NMA[i] + SD_up * iStdDev(NULL, 0, SD_len, 0, 0, PRICE_CLOSE, i); SDdn[i] = NMA[i] - SD_dn * iStdDev(NULL, 0, SD_len, 0, 0, PRICE_CLOSE, i); SD_Band_Osc[i] = (Close[i] - SDdn[i]) / (SDup[i] - SDdn[i]) * 100; } return(0); }
Â
The source code is also available for download here:
- C0UNDE, ahuramazdi, mashki and 7 others
-
10
-
NMA_SD_Band_Width for MT4
Â
NMA_SD_Band_Width.
Â
Though fully faithful to the original TradeStation source code, this one shouldn't be called NMA_anything as it has basically nothing to do with NMA. Pat probably didn't realize that he calculates the SD bands from the price, not from the NMA, and thus the difference between the upper and lower band is mere a sum of both deviation constants:
SDup = NMA + SD_up * StdDev SDdn = NMA - SD_dn * StdDev SDwidth = SDup - SDdn = NMA + SD_up * StdDev - (NMA - SD_dn * StdDev) = NMA - NMA + SD_up * StdDev + SD_dn * StdDev = (SD_up + SD_dn) * iStdDev
Â
EDIT: Pat actually said on the video that what this indicator does is measuring the volatility. I guess he just named it NMA_... for convenience. It's a simple idea but definitely not unuseful.
Â
Â
Original NMA_SD_Band_Width on TradeStation:
http://img339.imageshack.us/img339/3770/nmasdbandwidthtradestat.png
Â
NMA_SD_Band_Width on Metatrader:
http://img190.imageshack.us/img190/8113/nmasdbandwidthmetatrade.png
Â
Original size screenshots:
http://img853.imageshack.us/img853/3770/nmasdbandwidthtradestat.png
http://img189.imageshack.us/img189/8113/nmasdbandwidthmetatrade.png
Â
#property indicator_separate_window #property indicator_buffers 1 #property indicator_color1 Blue extern int NMA_period = 40; extern bool FastNMA = true; extern int NMA_LB_min = 8; extern int SD_len = 20; extern double SD_up = 1.5; extern double SD_dn = 1.5; extern bool Show_Pct = false; double SD_Band_Width[]; double NMA[]; double SDup[]; double SDdn[]; int init() { string nmaname = "NMA_SD_Band_Width(" + NMA_period + ")"; if(FastNMA) nmaname = "Fast_NMA_SD_Band_Width(" + NMA_period + ", " + NMA_LB_min + ")"; IndicatorShortName(nmaname); IndicatorBuffers(4); SetIndexBuffer(0, SD_Band_Width); SetIndexLabel(0, nmaname); SetIndexBuffer(1, NMA); SetIndexBuffer(2, SDup); SetIndexBuffer(3, SDdn); return(0); } int start() { int limit, i, ii, counted_bars = IndicatorCounted(); if(Bars <= MathMax(NMA_period, SD_len)) return(0); if(counted_bars < 0) counted_bars = 0; if(counted_bars > MathMax(NMA_period, SD_len)) limit = Bars - counted_bars; else limit = Bars - MathMax(NMA_period, SD_len) - 1; for(i = limit; i >= 0; i--) { double nmmnum, maxnmm = 0, sum, abssum, ratio = 0; int NMA_LB_max; if(FastNMA) { for(ii = 1; ii <= NMA_period; ii++) { nmmnum = (MathLog(Close[i]) - MathLog(Close[i+ii])) / MathSqrt(ii); if(MathAbs(nmmnum) > maxnmm) { maxnmm = MathAbs(nmmnum); NMA_LB_max = ii; } } if(NMA_LB_max < NMA_LB_min) NMA_LB_max = NMA_LB_min; } else NMA_LB_max = NMA_period; sum = (MathLog(Close[i]) - MathLog(Close[i+1])) + (MathLog(Close[i+1]) - MathLog(Close[i+2])) * (MathSqrt(2)-1); for(ii = 2; ii < NMA_LB_max; ii++) sum += (MathLog(Close[i+ii]) - MathLog(Close[i+ii+1])) * (MathSqrt(ii+1) - MathSqrt(ii)); abssum = MathAbs(sum); sum = 0; for(ii = 0; ii < NMA_LB_max; ii++) sum += MathAbs(MathLog(Close[i+ii]) - MathLog(Close[i+ii+1])); if(sum != 0) ratio = abssum / sum; NMA[i] = NMA[i+1] + (Close[i] - NMA[i+1]) * ratio; SDup[i] = NMA[i] + SD_up * iStdDev(NULL, 0, SD_len, 0, 0, PRICE_CLOSE, i); SDdn[i] = NMA[i] - SD_dn * iStdDev(NULL, 0, SD_len, 0, 0, PRICE_CLOSE, i); if(Show_Pct) SD_Band_Width[i] = (SDup[i] - SDdn[i]) / NMA[i] * 100; else SD_Band_Width[i] = SDup[i] - SDdn[i]; } return(0); }
Â
The source code is also available for download here:
-
NMM_X-Ray_2 for MT4
Â
NMM_X-Ray_2.
Â
There are two modes (switches) - "Show_value" and "Show_LB". They can be switched on both at the same time, although it's not recommended as they have different scales.
Â
In the "Show_LB" mode the scale is from 0 to 40 (no percentage option) and the same remark regarding the consequences of changing the "NMM_period" variable applies as with NMM_X-Ray_1.
Â
In the "Show_value" mode the chart's fixed minimum and maximum should be deactivated. I added zero dotted line to make the sign line (red) better recognizable.
Â
Â
Original NMM_X-Ray_2 on TradeStation:
http://img600.imageshack.us/img600/8945/nmmxray2tradestation.png
Â
NMM_X-Ray_2 on Metatrader:
http://img836.imageshack.us/img836/374/nmmxray2metatrader.png
Â
Original size screenshots:
http://img841.imageshack.us/img841/8945/nmmxray2tradestation.png
http://img209.imageshack.us/img209/374/nmmxray2metatrader.png
Â
#property indicator_separate_window #property indicator_minimum 0 #property indicator_maximum 40 #property indicator_buffers 3 #property indicator_color1 Red #property indicator_color2 Blue #property indicator_color3 Aqua #property indicator_level1 0 #property indicator_level2 20 #property indicator_levelcolor Gray #property indicator_levelstyle 2 extern int NMM_period = 40; extern bool Show_value = false; extern bool Show_LB = true; double NMMmaxratio[]; double NMMsign[]; double LBlen[]; int init() { string nmmname = "NMM_X-Ray_2"; IndicatorShortName(nmmname + "(" + NMM_period + ")"); if(Show_value) IndicatorDigits(2); else IndicatorDigits(0); IndicatorBuffers(3); SetIndexBuffer(0, NMMmaxratio); SetIndexLabel(0, nmmname + "(" + NMM_period + ")"); SetIndexBuffer(1, NMMsign); SetIndexLabel(1, nmmname + "_sign"); SetIndexBuffer(2, LBlen); SetIndexLabel(2, nmmname + "_LBlen"); return(0); } int start() { int limit, i, ii, counted_bars = IndicatorCounted(); if(Bars <= NMM_period) return(0); if(counted_bars < 0) counted_bars = 0; if(counted_bars > NMM_period) limit = Bars - counted_bars; else limit = Bars - NMM_period - 1; for(i = limit; i >= 0; i--) { double nmmnum, nmmmax = -1, nmmsign, nmmlb; for(ii = 1; ii <= NMM_period; ii++) { nmmnum = (MathLog(Close[i]) - MathLog(Close[i+ii])) / MathSqrt(ii); if(MathAbs(nmmnum) > nmmmax) { nmmmax = MathAbs(nmmnum); nmmlb = ii; if(nmmnum > 0) nmmsign = 1; else if(nmmnum < 0) nmmsign = -1; else nmmsign = 0; } } if(Show_value) { NMMmaxratio[i] = nmmmax * 1000; NMMsign[i] = nmmsign; } if(Show_LB) LBlen[i] = nmmlb; } return(0); }
Â
The source code is also available for download here:
-
NMM_X-Ray_1 for MT4
Â
NMM_X-Ray_1.
Â
The scale of this indicator is from 0 to 40 with a dotted auxiliary middle line at 20. The number 40 is hardwired in the original TradeStation's version, I made it changeable via external variable called "NMM_period". You have no real reason to change it but if you do then you should also change the scale and the middle line appropriately.
Â
Also, there is a switch Show_Pct, turned off by default, to show values in percentage (what was 0 - 40 would be 0 - 100). If you use that switch you should respectively adjust the scale and the middle line.
Â
The TradeStation's version shows only the PosCnt value (the chart line). I show all 3 values (PosCnt, NegCnt and ZeroCnt) where the two others have by default no color assigned (thus are not visible). You can assign them a color, or, you can check their values via "Data Window". All three most recent values are also always shown in the upper left corner of the indicator chart window.
Â
Â
Original NMM_X-Ray_1 on TradeStation:
http://img341.imageshack.us/img341/7481/nmmxray1tradestation.png
Â
NMM_X-Ray_1 on Metatrader:
http://img10.imageshack.us/img10/6563/nmmxray1metatrader.png
Â
Original size screenshots:
http://img829.imageshack.us/img829/7481/nmmxray1tradestation.png
http://img849.imageshack.us/img849/6563/nmmxray1metatrader.png
Â
#property indicator_separate_window #property indicator_minimum 0 #property indicator_maximum 40 #property indicator_buffers 3 #property indicator_color1 Yellow #property indicator_color2 CLR_NONE #property indicator_color3 CLR_NONE #property indicator_level1 20 #property indicator_levelcolor Gray #property indicator_levelstyle 2 extern int NMM_period = 40; extern bool Show_Pct = false; double NMM[]; double NegCnt[]; double ZeroCnt[]; int init() { string nmmname = "NMM_X-Ray_1"; IndicatorShortName(nmmname + "(" + NMM_period + ")"); IndicatorBuffers(3); SetIndexBuffer(0, NMM); SetIndexLabel(0, nmmname + "(" + NMM_period + ")"); SetIndexBuffer(1, NegCnt); SetIndexLabel(1, nmmname + "_NegCnt"); SetIndexBuffer(2, ZeroCnt); SetIndexLabel(2, nmmname + "_ZeroCnt"); return(0); } int start() { int limit, i, ii, counted_bars = IndicatorCounted(); if(Bars <= NMM_period) return(0); if(counted_bars < 0) counted_bars = 0; if(counted_bars > NMM_period) limit = Bars - counted_bars; else limit = Bars - NMM_period - 1; for(i = limit; i >= 0; i--) { double nmmnum, Pos = 0, Neg = 0, Zero = 0; for(ii = 1; ii <= NMM_period; ii++) { nmmnum = (MathLog(Close[i]) - MathLog(Close[i+ii])) / MathSqrt(ii); if(nmmnum > 0) Pos++; else if(nmmnum < 0) Neg++; else Zero++; } if(Show_Pct) { NMM[i] = Pos / NMM_period * 100; NegCnt[i] = Neg / NMM_period * 100; ZeroCnt[i] = Zero / NMM_period * 100; } else { NMM[i] = Pos; NegCnt[i] = Neg; ZeroCnt[i] = Zero; } } return(0); }
Â
The source code is also available for download here:
- mashki, ahuramazdi, for-ex and 6 others
-
9
Ocean Theory indis from TSD elite
in MetaTrader Indicators
Posted
Ocn_NMRx for MT4
Â
Ocn_NMRx.
Â
This poor one didn't even have NMAs, now it does :)
Â
Â
Â
The source code is also available for download here:
http://pastebin.com/eUTa0wTj