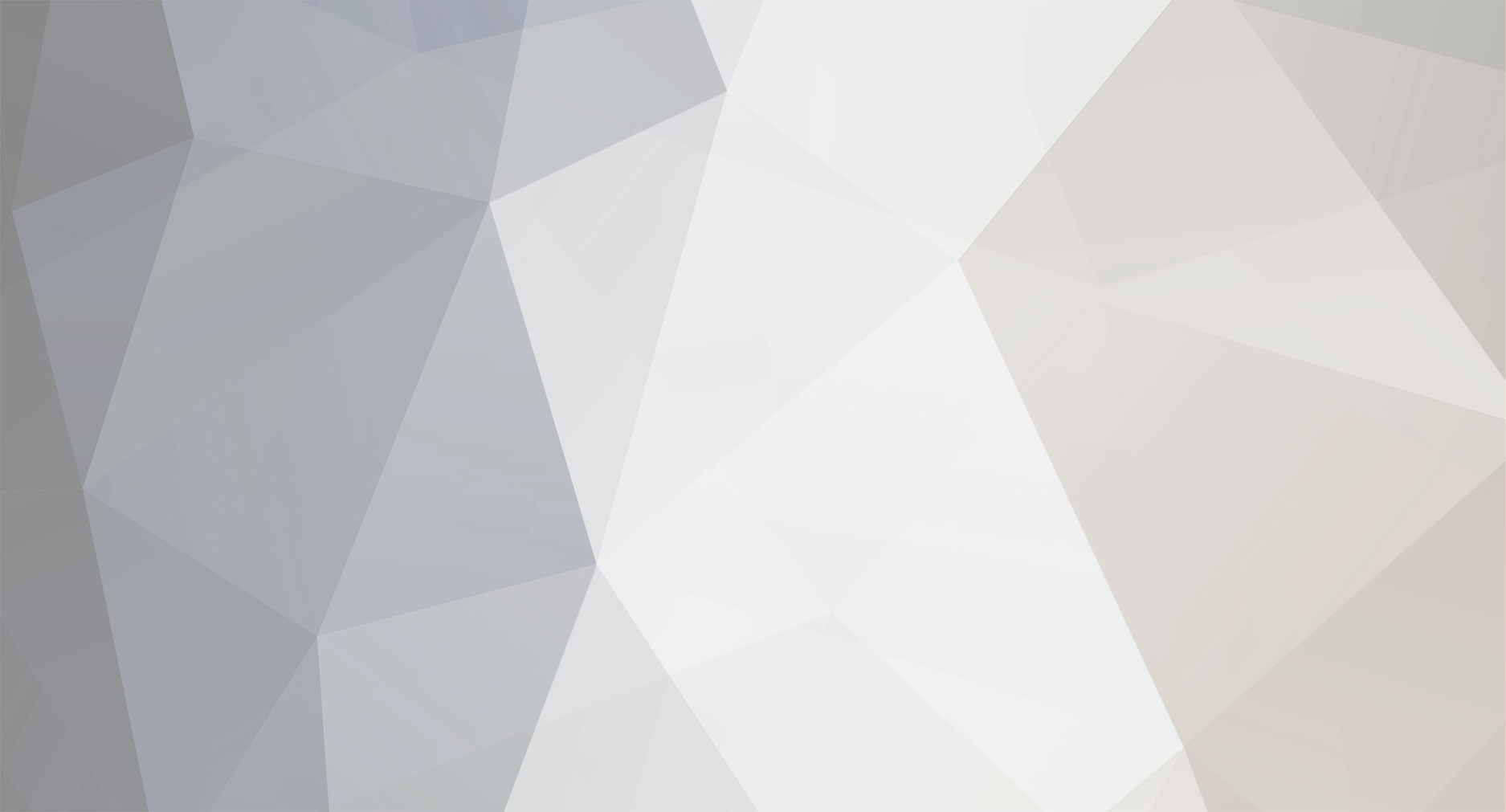
schnucki2801
-
Posts
14 -
Joined
-
Last visited
Content Type
Profiles
Forums
Articles
Posts posted by schnucki2801
-
-
-
Hi, can everyone edit this indicator for MT4, it's only for Ninja Trader. Many thanks for your help.
//
// Copyright © 2006, NinjaTrader LLC <[email protected]>.
// NinjaTrader reserves the right to modify or overwrite this NinjaScript component with each release.
//
#region Using declarations
using System;
using System.ComponentModel;
using System.Drawing;
using System.Drawing.Drawing2D;
using System.Xml.Serialization;
using NinjaTrader.Data;
using NinjaTrader.Gui.Chart;
#endregion
#region NinjaScript generated code. Neither change nor remove.
// This namespace holds all indicators and is required. Do not change it.
namespace NinjaTrader.Indicator
{
public partial class Indicator : IndicatorBase
{
private CCIDivV2[] cacheCCIDivV2 = null;
private static CCIDivV2 checkCCIDivV2 = new CCIDivV2();
/// <summary>
/// Enter the description of your new custom indicator here
/// </summary>
/// <returns></returns>
public CCIDivV2 CCIDivV2(int distance, int noOfBarsAfter, int noOfBarsBefore, int period, SoundCCI soundAlert, ProTypeCCIV2Dot term)
{
return CCIDivV2(Input, distance, noOfBarsAfter, noOfBarsBefore, period, soundAlert, term);
}
/// <summary>
/// Enter the description of your new custom indicator here
/// </summary>
/// <returns></returns>
public CCIDivV2 CCIDivV2(Data.IDataSeries input, int distance, int noOfBarsAfter, int noOfBarsBefore, int period, SoundCCI soundAlert, ProTypeCCIV2Dot term)
{
if (cacheCCIDivV2 != null)
for (int idx = 0; idx < cacheCCIDivV2.Length; idx++)
if (cacheCCIDivV2[idx].Distance == distance && cacheCCIDivV2[idx].NoOfBarsAfter == noOfBarsAfter && cacheCCIDivV2[idx].NoOfBarsBefore == noOfBarsBefore && cacheCCIDivV2[idx].Period == period && cacheCCIDivV2[idx].SoundAlert == soundAlert && cacheCCIDivV2[idx].Term == term && cacheCCIDivV2[idx].EqualsInput(input))
return cacheCCIDivV2[idx];
lock (checkCCIDivV2)
{
checkCCIDivV2.Distance = distance;
distance = checkCCIDivV2.Distance;
checkCCIDivV2.NoOfBarsAfter = noOfBarsAfter;
noOfBarsAfter = checkCCIDivV2.NoOfBarsAfter;
checkCCIDivV2.NoOfBarsBefore = noOfBarsBefore;
noOfBarsBefore = checkCCIDivV2.NoOfBarsBefore;
checkCCIDivV2.Period = period;
period = checkCCIDivV2.Period;
checkCCIDivV2.SoundAlert = soundAlert;
soundAlert = checkCCIDivV2.SoundAlert;
checkCCIDivV2.Term = term;
term = checkCCIDivV2.Term;
if (cacheCCIDivV2 != null)
for (int idx = 0; idx < cacheCCIDivV2.Length; idx++)
if (cacheCCIDivV2[idx].Distance == distance && cacheCCIDivV2[idx].NoOfBarsAfter == noOfBarsAfter && cacheCCIDivV2[idx].NoOfBarsBefore == noOfBarsBefore && cacheCCIDivV2[idx].Period == period && cacheCCIDivV2[idx].SoundAlert == soundAlert && cacheCCIDivV2[idx].Term == term && cacheCCIDivV2[idx].EqualsInput(input))
return cacheCCIDivV2[idx];
CCIDivV2 indicator = new CCIDivV2();
indicator.BarsRequired = BarsRequired;
indicator.CalculateOnBarClose = CalculateOnBarClose;
#if NT7
indicator.ForceMaximumBarsLookBack256 = ForceMaximumBarsLookBack256;
indicator.MaximumBarsLookBack = MaximumBarsLookBack;
#endif
indicator.Input = input;
indicator.Distance = distance;
indicator.NoOfBarsAfter = noOfBarsAfter;
indicator.NoOfBarsBefore = noOfBarsBefore;
indicator.Period = period;
indicator.SoundAlert = soundAlert;
indicator.Term = term;
Indicators.Add(indicator);
indicator.SetUp();
CCIDivV2[] tmp = new CCIDivV2[cacheCCIDivV2 == null ? 1 : cacheCCIDivV2.Length + 1];
if (cacheCCIDivV2 != null)
cacheCCIDivV2.CopyTo(tmp, 0);
tmp[tmp.Length - 1] = indicator;
cacheCCIDivV2 = tmp;
return indicator;
}
}
}
}
// This namespace holds all market analyzer column definitions and is required. Do not change it.
namespace NinjaTrader.MarketAnalyzer
{
public partial class Column : ColumnBase
{
/// <summary>
/// Enter the description of your new custom indicator here
/// </summary>
/// <returns></returns>
[Gui.Design.WizardCondition("Indicator")]
public Indicator.CCIDivV2 CCIDivV2(int distance, int noOfBarsAfter, int noOfBarsBefore, int period, SoundCCI soundAlert, ProTypeCCIV2Dot term)
{
return _indicator.CCIDivV2(Input, distance, noOfBarsAfter, noOfBarsBefore, period, soundAlert, term);
}
/// <summary>
/// Enter the description of your new custom indicator here
/// </summary>
/// <returns></returns>
public Indicator.CCIDivV2 CCIDivV2(Data.IDataSeries input, int distance, int noOfBarsAfter, int noOfBarsBefore, int period, SoundCCI soundAlert, ProTypeCCIV2Dot term)
{
return _indicator.CCIDivV2(input, distance, noOfBarsAfter, noOfBarsBefore, period, soundAlert, term);
}
}
}
// This namespace holds all strategies and is required. Do not change it.
namespace NinjaTrader.Strategy
{
public partial class Strategy : StrategyBase
{
/// <summary>
/// Enter the description of your new custom indicator here
/// </summary>
/// <returns></returns>
[Gui.Design.WizardCondition("Indicator")]
public Indicator.CCIDivV2 CCIDivV2(int distance, int noOfBarsAfter, int noOfBarsBefore, int period, SoundCCI soundAlert, ProTypeCCIV2Dot term)
{
return _indicator.CCIDivV2(Input, distance, noOfBarsAfter, noOfBarsBefore, period, soundAlert, term);
}
/// <summary>
/// Enter the description of your new custom indicator here
/// </summary>
/// <returns></returns>
public Indicator.CCIDivV2 CCIDivV2(Data.IDataSeries input, int distance, int noOfBarsAfter, int noOfBarsBefore, int period, SoundCCI soundAlert, ProTypeCCIV2Dot term)
{
if (InInitialize && input == null)
throw new ArgumentException("You only can access an indicator with the default input/bar series from within the 'Initialize()' method");
return _indicator.CCIDivV2(input, distance, noOfBarsAfter, noOfBarsBefore, period, soundAlert, term);
}
}
}
#endregion
-
pls reupload, the indi doesn't work
-
It is appear and cost $87. But with Plimus I don't buy.
-
Hi all,
can anyony change this script that input for SL and TP is a price and not in pips. Many thanks for your help.
Sorry for my bad English but I hope you understand what I mean.
-
-
You're great man! Thank you! Just a question.. Do you have the versions SA-204_Golden Upgrade and SA-207 Ultimate Upgrade too? :)
No, sorry, I'm only have this version
-
-
Hi monik7,
great work. You should insert delete opposite pending order if open an order and delete pending orders after 4 hours.
Sorry for my bad English. I hope you understand what I mean.
-
-
Can everyone share?
-
-
Hi all,
Does anyone have this EA?
hxxp://xxx.forexultrabot.com/
Please use code display when posting a link
SF
Forex Trend Champion
in MetaTrader Indicators
Posted
Hi all, does someone have this indicator? It looks good. Please share.
http://www.forextrendchampion.com/