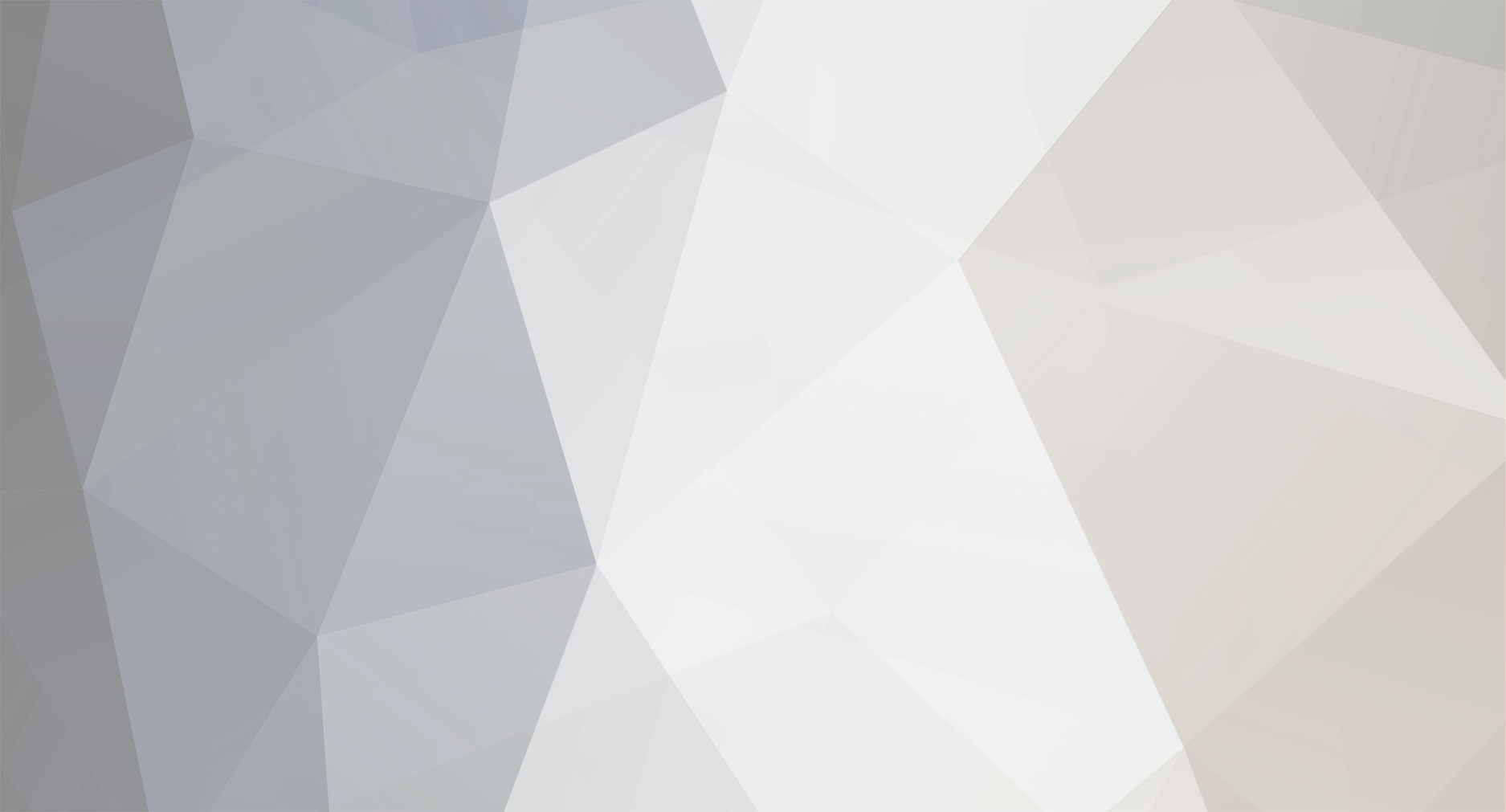
posterx
-
Posts
19 -
Joined
-
Last visited
Content Type
Profiles
Forums
Articles
Posts posted by posterx
-
-
Hello,
I am looking for this course : http://trading.bkforex.com/trendcatcher-strategy/
Anyone has it..please share.
Thanks in advance
-
I am getting the following errors when compiling the MT4 code. Can anyone please help.
Thanks
http://img249.imageshack.us/img249/6641/adxerror.jpg
-
Hello,
The ADX indicator, Wilders version is missing from MT4 but available with MT5. Below is the MT5 code.
Can any MT coding expert please port this code to MT4.
Many Thanks
//+------------------------------------------------------------------+
//| ADXW.mq5 |
//| Copyright 2009, MetaQuotes Software Corp. |
//| http://www.mql5.com |
//+------------------------------------------------------------------+
#property copyright "2009, MetaQuotes Software Corp."
#property link "http://www.mql5.com"
#property description "Average Directional Movement Index"
#property description "by Welles Wilder"
#include <MovingAverages.mqh>
//---
#property indicator_separate_window
#property indicator_buffers 10
#property indicator_plots 3
#property indicator_type1 DRAW_LINE
#property indicator_style1 STYLE_SOLID
#property indicator_width1 1
#property indicator_color1 LightSeaGreen
#property indicator_type2 DRAW_LINE
#property indicator_style2 STYLE_DOT
#property indicator_width2 1
#property indicator_color2 YellowGreen
#property indicator_type3 DRAW_LINE
#property indicator_style3 STYLE_DOT
#property indicator_width3 1
#property indicator_color3 Wheat
#property indicator_label1 "ADX Wilder"
#property indicator_label2 "+DI"
#property indicator_label3 "-DI"
//--- input parameters
input int InpPeriodADXW=14; // Period
//---- buffers
double ExtADXWBuffer[];
double ExtPDIBuffer[];
double ExtNDIBuffer[];
double ExtPDSBuffer[];
double ExtNDSBuffer[];
double ExtPDBuffer[];
double ExtNDBuffer[];
double ExtTRBuffer[];
double ExtATRBuffer[];
double ExtDXBuffer[];
//--- global variable
int ExtADXWPeriod;
//+------------------------------------------------------------------+
//| Custom indicator initialization function |
//+------------------------------------------------------------------+
void OnInit()
{
//--- check for input parameters
if(InpPeriodADXW>=100 || InpPeriodADXW<=0)
{
ExtADXWPeriod=14;
printf("Incorrect value for input variable InpPeriodADXW=%d. Indicator will use value=%d for calculations.",InpPeriodADXW,ExtADXWPeriod);
}
else ExtADXWPeriod=InpPeriodADXW;
//---- indicator buffers
SetIndexBuffer(0,ExtADXWBuffer);
SetIndexBuffer(1,ExtPDIBuffer);
SetIndexBuffer(2,ExtNDIBuffer);
//--- calculation buffers
SetIndexBuffer(3,ExtPDBuffer,INDICATOR_CALCULATIONS);
SetIndexBuffer(4,ExtNDBuffer,INDICATOR_CALCULATIONS);
SetIndexBuffer(5,ExtDXBuffer,INDICATOR_CALCULATIONS);
SetIndexBuffer(6,ExtTRBuffer,INDICATOR_CALCULATIONS);
SetIndexBuffer(7,ExtATRBuffer,INDICATOR_CALCULATIONS);
SetIndexBuffer(8,ExtPDSBuffer,INDICATOR_CALCULATIONS);
SetIndexBuffer(9,ExtNDSBuffer,INDICATOR_CALCULATIONS);
//--- set draw begin
PlotIndexSetInteger(0,PLOT_DRAW_BEGIN,ExtADXWPeriod<<1);
PlotIndexSetInteger(1,PLOT_DRAW_BEGIN,ExtADXWPeriod+1);
PlotIndexSetInteger(2,PLOT_DRAW_BEGIN,ExtADXWPeriod+1);
//--- indicator short name
string short_name="ADX Wilder("+string(ExtADXWPeriod)+")";
IndicatorSetString(INDICATOR_SHORTNAME,short_name);
//--- change 1-st index label
PlotIndexSetString(0,PLOT_LABEL,short_name);
//--- indicator digits
IndicatorSetInteger(INDICATOR_DIGITS,2);
//---- end of initialization function
}
//+------------------------------------------------------------------+
//| Custom indicator iteration function |
//+------------------------------------------------------------------+
int OnCalculate(const int rates_total,const int prev_calculated,
const datetime &Time[],
const double &Open[],
const double &High[],
const double &Low[],
const double &Close[],
const long &TickVolume[],
const long &Volume[],
const int &Spread[])
{
//--- checking for bars count
if(rates_total<ExtADXWPeriod)
return(0);
//--- detect start position
int start;
if(prev_calculated>1) start=prev_calculated-1;
else
{
start=1;
for(int i=0;i<ExtADXWPeriod;i++)
{
ExtADXWBuffer=0;
ExtPDIBuffer=0;
ExtNDIBuffer=0;
ExtPDSBuffer=0;
ExtNDSBuffer=0;
ExtPDBuffer=0;
ExtNDBuffer=0;
ExtTRBuffer=0;
ExtATRBuffer=0;
ExtDXBuffer=0;
}
}
//--- main cycle
for(int i=start;i<rates_total;i++)
{
//--- get some data
double Hi =High;
double prevHi=High[i-1];
double Lo =Low;
double prevLo=Low[i-1];
double prevCl=Close[i-1];
//--- fill main positive and main negative buffers
double dTmpP=Hi-prevHi;
double dTmpN=prevLo-Lo;
if(dTmpP<0.0) dTmpP=0.0;
if(dTmpN<0.0) dTmpN=0.0;
if(dTmpN==dTmpP)
{
dTmpN=0.0;
dTmpP=0.0;
}
else
{
if(dTmpP<dTmpN) dTmpP=0.0;
else dTmpN=0.0;
}
ExtPDBuffer=dTmpP;
ExtNDBuffer=dTmpN;
//--- define TR
double tr=MathMax(MathMax(MathAbs(Hi-Lo),MathAbs(Hi-prevCl)),MathAbs(Lo-prevCl));
//--- write down TR to TR buffer
ExtTRBuffer=tr;
//--- fill smoothed positive and negative buffers and TR buffer
if(i<ExtADXWPeriod)
{
ExtATRBuffer=0.0;
ExtPDIBuffer=0.0;
ExtNDIBuffer=0.0;
}
else
{
ExtATRBuffer=SmoothedMA(i,ExtADXWPeriod,ExtATRBuffer[i-1],ExtTRBuffer);
ExtPDSBuffer=SmoothedMA(i,ExtADXWPeriod,ExtPDSBuffer[i-1],ExtPDBuffer);
ExtNDSBuffer=SmoothedMA(i,ExtADXWPeriod,ExtNDSBuffer[i-1],ExtNDBuffer);
}
//--- calculate PDI and NDI buffers
if(ExtATRBuffer!=0.0)
{
ExtPDIBuffer=100.0*ExtPDSBuffer/ExtATRBuffer;
ExtNDIBuffer=100.0*ExtNDSBuffer/ExtATRBuffer;
}
else
{
ExtPDIBuffer=0.0;
ExtNDIBuffer=0.0;
}
//--- Calculate DX buffer
double dTmp=ExtPDIBuffer+ExtNDIBuffer;
if(dTmp!=0.0) dTmp=100.0*MathAbs((ExtPDIBuffer-ExtNDIBuffer)/dTmp);
else dTmp=0.0;
ExtDXBuffer=dTmp;
//--- fill ADXW buffer as smoothed DX buffer
ExtADXWBuffer=SmoothedMA(i,ExtADXWPeriod,ExtADXWBuffer[i-1],ExtDXBuffer);
}
//---- OnCalculate done. Return new prev_calculated.
return(rates_total);
}
//+------------------------------------------------------------------+
-
Hello Guys,
Please find below most of the videos from Strategy Depot which is a forex video training website.
hxxp://www.megaupload.com/?d=V12Q9Y3U
Their latest addition is an EA. Check link below. As per the videos, its an awesome EA. 2000+ pips in 1 month!!!
http://www.strategydepot.com/amazingprofits.php
Anyone has the EA, please share.
Thanks
-
Re: Futures Uncovered System
can someone post a rs mirror? always have problem downloading from megauploadhxxp://r@[email protected]/files/264269845/Futures_Uncovered.zip
-
Re: Futures Uncovered System
the video links do not openuseless without them.
thanks
They do run. They are embedded into the pdf file itself. Use the latest adobe reader. They do run fine on my computer
-
Re: Mark Douglas Psychology of Trading Audio
Thanks!!!!!!!!!
-
Re: Scalping...is this a waste of time or can you....
I don't believe that it's possible to do scalping without having a robot..It's just too time consuming to watch the trades.
You can't even put a SL and TP in the trade as it will be to close with your price
and most brokers will reject your SL and TP points.
Many trends start at around the start of the London session. If you monitor at this time, you can be sure to catch some decent pips. This is especially true for GBPUSD and EURUSD.
-
Re: Futures Uncovered System
love to view it.. please give link.The link is already in the post...
-
Hello Guys,
Just want to share something on this great forum. Below is the link to download the Futures Uncovered trading system. It consists of 2 PDF files both needing a password to open.
The password is
For PDF 1: t997xr8pq
For PDF 2: 93rmp7s77
http://www.megaupload.com/?d=573IA3OC
or
hxxp://r@[email protected]/files/264269845/Futures_Uncovered.zip
-
Re: Scalping...is this a waste of time or can you....
Posterx, can i know the entry and exit rules for your system?
Btw it's on TF 15 Minutes rite?
Ok.The 10/21 crossover will give buy/sell signals. MACD should be crossing above/below 0 level. ADX should be rising above 20 for strong trend.
If you have missed the crossover signal, wait for a retrace to 10/21 EMA. Usually most powerful trends do retrace at least to the 10EMA. Then you enter.
The exit signal can be a break of the 21EMA or better, an opposite crossover.
I use this 10Min system in conjunction with a 1HR system to get the broader picture.
-
Re: EA : PIPTURBO By Carter Stevenson
I am demo testing Pipturbo on FxSolutions.com practice account. Their MT4 uses EST. I am not sure of the OpenHour and CloseHour settings.
EST = GMT -4
As per the settings manual, it should be but the CloseHour will be -ve!!!
OpenHour = 21 - 4 = 17
CloseHour = 3 - 4 = -1?????
Anybody can help with this??
Thxxx
-
Re: EA : PIPTURBO By Carter Stevenson
15 Min EURUSD, EURCHF, USDJPY, GBPUSD and USDCHF
-
Re: How Detrmine if Market is Trading Sideways?
If you use Moving Averages, they will be flat. Also, ADX will be below 20. At times, a higher time frame can help as well.
-
Re: EA : PIPTURBO By Carter Stevenson
What timeframe does it work on????
-
Re: When You First Entered The Forex World . . .
Once you have a strategy in place, don't wait too long. Open a live account with small money. Trading demo and live is very different. There are no emotions with demo.
-
Re: Scalping...is this a waste of time or can you....
Try 10/21 EMA on 10Min chart with MACD settings (5,13,1) and ADX (14). It works great.
-
Re: Help starting with Forex
Making money in forex is not an easy task. It takes years to become a consistent trader. Don't trade when you have money issues. Find another job. When your situation gets better, then trade. You can learn forex though, but don't rely on it for now.
Forex Flex EA
in MetaTrader Expert Advisors Request
Posted
Hello,
Anyone has forex flex ea (http://forexflexea.com/), please share.
Thanks