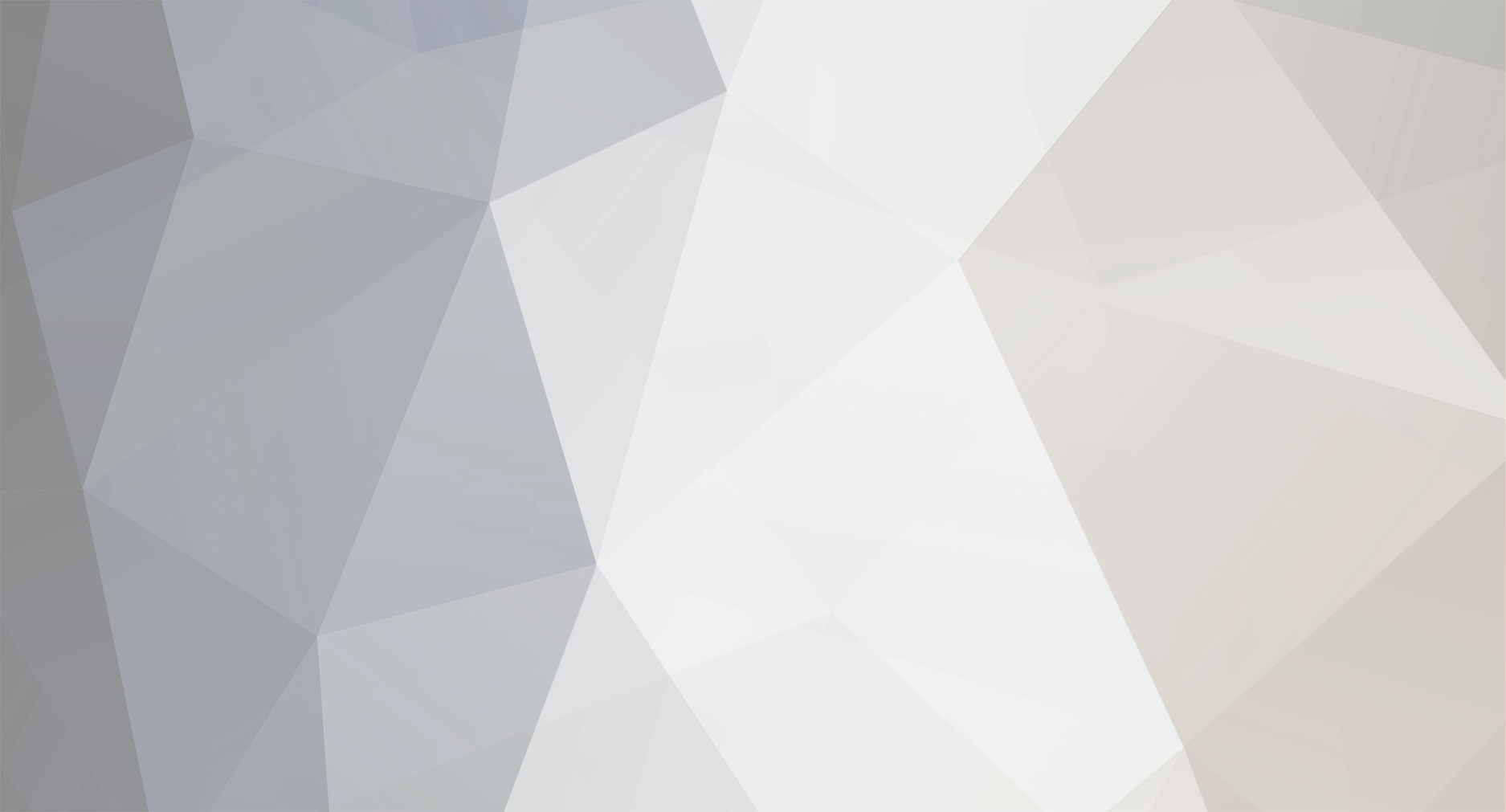
tongtoro
-
Posts
251 -
Joined
-
Last visited
-
Days Won
2
Content Type
Profiles
Forums
Articles
Posts posted by tongtoro
-
-
you can use this tempalte
//+------------------------------------------------------------------+
//| TangoPivotEA.mq4 |
//+------------------------------------------------------------------+
#property copyright "[email protected]"
#property link ""
extern int Delta1 = 5; // minimalna daljina close prehodnog dana od cppa novog dana
extern int Delta2 = 100; // maximalna -"-
extern double Lot = 0.01;
int MagicniBroj=1;
double VrednostPipa;
double AccountEquityPocetni;
double AccountBalancePocetni;
int decimala;
int BE=30;
int init() {
VrednostPipa = VrednostPipa(Symbol());
decimala=MarketInfo(Symbol(),MODE_DIGITS);
AccountEquityPocetni = AccountEquity();
return(0);
}
//+------------------------------------------------------------------+
//| expert start function |
//+------------------------------------------------------------------+
int start() {
if (iVolume(NULL,PERIOD_M15,0)==1) {
BEnaPipa();
}
if (iVolume(NULL,PERIOD_D1,0)==1) {
double DHigh, DLow, DClose;
double R3,R2,R1,CPP,S1,S2,S3,S4,S5,R4,R5,mr1,ms1;
double TPBuy1,TPBuy2,TPBuy3,TPSell1,TPSell2,TPSell3;
double SLBuy,SLSell;
int ticket;
DHigh = iHigh(NULL,PERIOD_D1,1);
DLow = iLow(NULL,PERIOD_D1,1);
DClose = iClose(NULL,PERIOD_D1,1);
CPP = NormalizeDouble(((DHigh + DLow + DClose)/3),decimala);
if (DayOfWeek()==0 && TimeDayOfWeek(Close[1])==5) { // ako smo u nedelji a prethodna sveca je petak
double H = iHigh(NULL,PERIOD_D1,1);
double L = iLow(NULL,PERIOD_D1,1);
double CLOSE = iClose(NULL,PERIOD_D1,1);
} else if (DayOfWeek()==1 && TimeDayOfWeek(Close[1])==0 && TimeDayOfWeek(Close[2])==5) { // ako smo u ponedeljku a prethodna sveca je nedelja
H = iHigh(NULL,PERIOD_D1,2);
L = iLow(NULL,PERIOD_D1,2);
CLOSE = iClose(NULL,PERIOD_D1,2);
} else { // ostalih dana uzmi prethodni dan
H = iHigh(NULL,PERIOD_D1,1);
L = iLow(NULL,PERIOD_D1,1);
CLOSE = iClose(NULL,PERIOD_D1,1);
}
// STANDARD PIVOTS
R1 = (2*CPP)-DLow;
S1 = (2*CPP)-DHigh;
R2 = CPP+(R1-S1);
S2 = CPP-(R1-S1);
R3 = (2.0*CPP) + (DHigh-(2.0*DLow));
S3 = (2.0*CPP) - ((2.0*DHigh)-DLow);
mr1 = (R1+CPP)/2;
ms1 = (S1+CPP)/2;
TPBuy1=NormalizeDouble(R1,decimala);
TPBuy2=NormalizeDouble(R2,decimala);
TPBuy3=NormalizeDouble(R3,decimala);
TPSell1=NormalizeDouble(S1,decimala);
TPSell2=NormalizeDouble(S2,decimala);
TPSell3=NormalizeDouble(S3,decimala);
SLBuy = NormalizeDouble(S1-0*VrednostPipa,decimala);
SLSell = NormalizeDouble(R1+0*VrednostPipa,decimala);
BE = MathRound((R2-CPP)/VrednostPipa);
int Isticanje = TimeCurrent()+16*3600; // ima smisla trejd do 16h popodne
// na pocetku novog dana redjamo pendinge ako imamo zeljenu razliku izmedju Close i novog CPP, pending stavljamo na cpp racunamo vratice se do njega
if(CPP-DClose>Delta1*VrednostPipa && CPP-DClose<Delta2*VrednostPipa){
ticket = OrderSend(Symbol(),OP_SELLLIMIT, Lot, CPP, 3, SLSell, TPSell1, "",MagicniBroj,Isticanje,Red);
if (AccountBalance()>AccountBalancePocetni) {
ticket = OrderSend(Symbol(),OP_SELLLIMIT, Lot, CPP, 3, SLSell, TPSell2, "",MagicniBroj,Isticanje,Red);
if (AccountBalance()>2*AccountBalancePocetni) {
ticket = OrderSend(Symbol(),OP_SELLLIMIT, Lot, CPP, 3, SLSell, TPSell3, "",MagicniBroj,Isticanje,Red);
}
}
}
if(DClose-CPP>Delta1*VrednostPipa && DClose-CPP<Delta2*VrednostPipa){
ticket = OrderSend(Symbol(),OP_BUYLIMIT, Lot, CPP, 3, SLBuy, TPBuy1, "",MagicniBroj,Isticanje,Green);
if (AccountBalance()>AccountBalancePocetni) {
ticket = OrderSend(Symbol(),OP_BUYLIMIT, Lot, CPP, 3, SLBuy, TPBuy2, "",MagicniBroj,Isticanje,Green);
if (AccountBalance()>2*AccountBalancePocetni) {
ticket = OrderSend(Symbol(),OP_BUYLIMIT, Lot, CPP, 3, SLBuy, TPBuy3, "",MagicniBroj,Isticanje,Green);
}
}
}
}
}
//+------------------------------------------------------------------+
double VrednostPipa(string Par) {
int decimala = MarketInfo(Par,MODE_DIGITS);
if (decimala==2 || decimala==3) double vrednostpipa = 0.01;
if (decimala==4 || decimala==5) vrednostpipa = 0.0001;
return (vrednostpipa);
}
void BEnaPipa() {
if (BE>0) {
// ako imamo BR profita, stavljamo na be
for (int i = OrdersTotal() - 1; i >= 0; i--) {
OrderSelect(i, SELECT_BY_POS, MODE_TRADES);
if (OrderSymbol() == Symbol() && OrderMagicNumber() == MagicniBroj) {
if (OrderType() == OP_SELL) {
//Print(OrderTicket()," ",OrderProfit()/OrderLots()/10 ," ",BE," ",OrderLots());
// ako je profit veci od BE i ako je sl iznad open price, stavljamo sl na op
if (OrderProfit()/OrderLots()/10 >= BE && OrderStopLoss() > OrderOpenPrice()) {
OrderModify(OrderTicket(), OrderOpenPrice(), OrderOpenPrice(), OrderTakeProfit(), 0, Red);
}
}
if (OrderType() == OP_BUY) {
// ako je profit veci od BE i ako je sl ispod open price, stavljamo sl na op
if (OrderProfit()/OrderLots()/10 >= BE && OrderStopLoss() < OrderOpenPrice()) {
OrderModify(OrderTicket(), OrderOpenPrice(), OrderOpenPrice(), OrderTakeProfit(), 0, Green);
}
}
}
}
}
}
-
Pippo: a Murrey Maths retrace auto-trading EA by SteveHopwood
-
wow.. this one looks interesting...
enjoy..
http://www.4shared.com/dir/jchkfhxW/carigold.html
-
-
-
Are yez sure like you're talkin 'bout the same EA here now? I can see these parameters here like now.
Symbol 2, period, period comparison, averaging, stop marga, Mon Delta, Step Delta, Lot, Lot 2, Magic (number), Order Total, N Open orders. I aint seen nothin' like you've got there there bein all that we're on the same planet now?
maybe different. i think so too.
i got from description button (hedge).
i am foward test on my vps now.
result in here http://x2t.com/Tongtoro
try with default setting. let see .......
-
Description of strategy
The idea of the strategy.
When the sign of the rate of price change to open a position in the direction of price changes.
The input parameters.
T1 - The value of the period;
b1 - The ratio of weight to the last value of the first to determine the rate of price change;
Pmin - the minimum value of current earnings, in which the stop loss moved to breakeven;
KTp - linear coefficient for setting take profit;
KSl-linear coefficient to set the stop loss;
SL0 - deviation set the stop loss from the opening price;
Tp0 - a free member to set a take profit;
Lot - lot size.
The calculated parameters
K1 i - rate of change of prices;
The opening position.
The position on the purchase of the i-th bar opens when the conditions K i-2 <0 and K i-1> 0 at the price of opening a bar. The position on the open market when the conditions K i-2> 0 and K i-1 <0 at the price of opening a bar. The opening position is, if there are no other open positions.
To calculate the magnitude of the velocity on the i-th bar, K i, you must first determine the weight bar p i from 1 to T1, where the first index corresponds to the i-T1 +1- th bar, and T1 - i-th by the formula:
XXXXXXXXXXXXXXXXXX
The value of K1 is defined by the formula (4):
XXXXXXXXXXXXXXX (2)
where
image (3)
image (4)
image (5)
image (6)
P i - the closing price of timeframes;
Ntf - the number of bars in a day.
At the opening of the position are set take profit and stop loss.
The value of take profit shifting from the opening price of the transaction on the value of D P Tp, which is determined by the formula:
image (7)
The value of stop loss is shifted from the opening price of the transaction on the value of SL0.
Changing the take profit and stop loss.
At each bar, take profit value is shifted from the opening price of the bar on value, calculated by the formula (7), if the displacement of more than take profit on the previous bar. Otherwise, take profit is unchanged.
The magnitude of the initial stop loss is changed only if the profit in pips for the current transaction is not less than Pmin. Then stop loss moves from the opening price of the transaction on the value of D P SL, which is determined by the formula:
image And (9)
where P op-opening price of the bar; P op.cl-opening price of the transaction.
In the future, stop loss is changed only if the value of D P SL on the current bar exceeds the previous value. The deal closed only take profit or stop loss.
-
Please download it from here, THANKS! n thanks to Pro!
Thanks man....
http://indo-investasi.com/showthread.php/12756-cmillion-Hedge
-
hi.
i just interest with the strategy. perhaps i can get the indicator
-
-
some body have this file
http://www.personal.reading.ac.uk/~sis01xh/teaching/CY2D2/Pattern1.pdfhttp://www.personal.reading.ac.uk/~sis01xh/teaching/CY2D2/Pattern2.pdf
http://www.personal.reading.ac.uk/~sis01xh/teaching/CY2D2/Pattern3.pdf
http://www.personal.reading.ac.uk/~sis01xh/teaching/CY2D2/Pattern4.pdf
http://www.personal.reading.ac.uk/~sis01xh/teaching/CY2D2/Pattern5.pdf
http://www.personal.reading.ac.uk/~sis01xh/teaching/CY2D2/Pattern6.pdf
http://www.personal.reading.ac.uk/~sis01xh/teaching/CY2D2/Pattern7.pdf
http://www.personal.reading.ac.uk/~sis01xh/teaching/CY2D2/Pattern8.pdf
http://www.personal.reading.ac.uk/~sis01xh/teaching/CY2D2/Pattern9.pdf"
Upload Please
Thanks
-
Does any body have MTS "HEDGE" from cmillion
Please share ...
Thanks
-
-
Thanks for the quantum ea
-
yes. it's useless. go to Foward Test
-
many thanks for Uploader
-
-
it's easy.
post your ea. i will add news filter just 1minuts
-
Upload again. please
-
Hi thanks
nice share
-
Hi
can u share dss Bressert indicator.
thanks
-
envivid
http://championship.mql5.com/2011/en/users/enivid/discussion
Xupypr
http://www.mql5.com/en/code/draft/611
you can find more mql5.com
-
What's mean steath?? Hidden SL & TP Or What,
if same with Hidden SL & TP, you can find trade manager
example MTPM (Forex factory) or Swiss army,
-
he try to tricky some one..
Looking for EA entering on Pivot R3 and S3 Level
in MetaTrader Expert Advisors Request
Posted
or
//+------------------------------------------------------------------+
//| Daily Pivot Point Trader.mq4 |
//| Copyright © 2011, Alan R Phillips |
//| http://www.forexfactory.com/showthread.php?t=330992 |
//+------------------------------------------------------------------+
#property copyright "Copyright © 2011, Alan R Phillips"
#property link ""
extern bool EAOn = false;
extern int TimeZone = 0; //currently doesn't do anything
extern double LotSize = 0.1;
extern int TakeProfitTarget = 0;
// 3,2,1 are R3/S3,R2/S2,R1/S1. 0 is no TP
//+------------------------------------------------------------------+
//| expert initialization function |
//+------------------------------------------------------------------+
int init()
{
//----
//----
return(0);
}
//+------------------------------------------------------------------+
//| expert deinitialization function |
//+------------------------------------------------------------------+
int deinit()
{
//----
//----
return(0);
}
//+------------------------------------------------------------------+
//| expert start function |
//+------------------------------------------------------------------+
int start()
{
double DHigh, DLow, DClose;
double R3,R2,R1,Pivot,S1,S2,S3;
string EASymbol;
double TPTargetBuy = 0.0;
double TPTargetSell = 0.0;
int ticket;
DHigh = NormalizeDouble(iHigh(NULL,PERIOD_D1,1),4);
DLow = NormalizeDouble(iLow(NULL,PERIOD_D1,1),4);
DClose = NormalizeDouble(iClose(NULL,PERIOD_D1,1),4);
Pivot = NormalizeDouble(((DHigh + DLow + DClose)/3),4);
R1 = (2*Pivot)-DLow;
S1 = (2*Pivot)-DHigh;
R2 = Pivot+(R1-S1);
S2 = Pivot-(R1-S1);
R3 = ( 2.0 * Pivot) + ( DHigh - ( 2.0 * DLow ) );
S3 = ( 2.0 * Pivot) - ( ( 2.0 * DHigh ) - DLow );
EASymbol = Symbol();
if(TakeProfitTarget == 0)
{
TPTargetBuy = 0.0;
TPTargetSell = 0.0;
}
if(TakeProfitTarget == 1)
{
TPTargetBuy = R1;
TPTargetSell = S1;
}
if(TakeProfitTarget == 2)
{
TPTargetBuy = R2;
TPTargetSell = S2;
}
if(TakeProfitTarget == 3)
{
TPTargetBuy = R3;
TPTargetSell = S3;
}
if(EAOn == True)
{
if( DClose < Pivot)
{
ticket = OrderSend(EASymbol,3, LotSize, Pivot, 0, R1, TPTargetSell, "Opened by DPP Trader",0,0,Blue);
Print(ticket);
}
if(DClose > Pivot)
{
ticket = OrderSend(EASymbol,2, LotSize, Pivot, 0, S1, TPTargetBuy, "Opened by DPP Trader",0,0,Blue);
Print(ticket);
}
}
else
{
Print("DPP Trader is off.");
}
return(0);
}